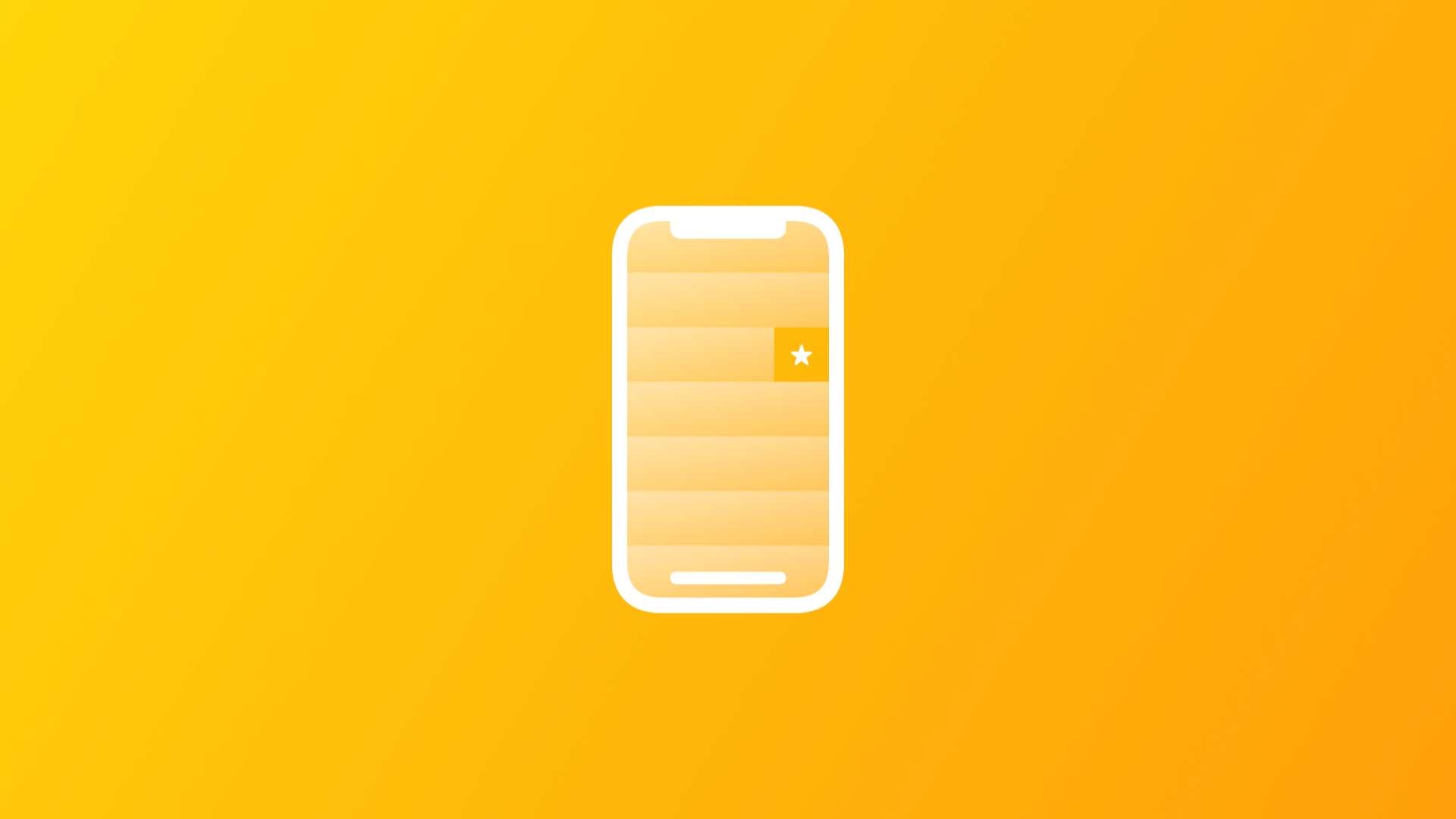
Swipe Actions on Lists with SwiftUI
By the end of this article, you will be able to add swipe actions to your lists using SwiftUI and new modifiers introduced at WWDC 2021.
One of the great new features added to SwiftUI on WWDC 2021 was the addition of a simple way to add swipe actions to your lists. With a simple modifier, you can add custom actions to your list items.
Let’s start by creating a simple list of names.
struct ContentView: View {
var contacts: [String] = ["Tiago Pereira", "Moritz Recke", "Giovanni Monaco"]
var body: some View {
// Creating a simple list of names
List(contacts, id: \.self) { name in
Text(name)
}
}
}
A simple list to be used in the examples that follow.
Now, let's see how to add swipe actions.
To add a swipe action to the elements of the list you just need to use the .swipeActions()
modifier on the list elements to add one or more actions to them, like in the example below.
struct ContentView: View {
var contacts: [String] = ["Tiago Pereira", "Moritz Recke", "Giovanni Monaco"]
var body: some View {
List(contacts, id: \.self) { name in
// Adding a swipe action to the items of the list.
Text(name)
.swipeActions {
Button {
print("\(name) is a favorite!")
} label: {
Label("Favorite", systemImage: "star")
}
.tint(.yellow)
}
}
}
}
Adding a swipe action to a list item by using the .swipeActions() modifier.
Remember to tint the button using the .tint modifier with a color related to the action it performs. You can also add a role to the button to customize its appearance. You can even use conditionals to change the label of the Button to match the data somehow, like marking an email as unread or read.
Now let’s take a look at different ways you can customize these actions in your application.
First, you can define on which side of the list item you want the actions to show up by telling which edge that particular action should show up at, .leading
or .trailing
.
Text(name)
.swipeActions(edge: .leading) {
Button {
print("\(item) is a favorite!")
} label: {
Label("Favorite", systemImage: "star")
}
.tint(.yellow)
}
Defining on which side of the list item the action will be placed.
Also, the full swipe behavior, in which if the user does a full swipe on your list item the action is triggered, is enabled by default, but if you want to disable it you can by setting the parameter allowFullSwipe
to false
.
Text(name)
.swipeActions(edge: .leading, allowFullSwipe: false) {
Button {
print("\(item) is a favorite!")
} label: {
Label("Favorite", systemImage: "star")
}
.tint(.yellow)
}
Turning off the default full swipe behavior.
You can also add multiple swipe actions to a list item just by using the modifier again.
Text(name)
.swipeActions(edge: .leading) {
Button {
print("\(name) is a favorite!")
} label: {
Label("Favorite", systemImage: "star")
}
.tint(.yellow)
}
.swipeActions(edge: .trailing, allowsFullSwipe: false) {
Button(role: .destructive) {
print("\(name) is being deleted.")
} label: {
Label("Delete", systemImage: "trash")
}
}
Adding multiple swipe actions to a list item.
You can check more about swipe actions on the official Apple Developer Documentation.
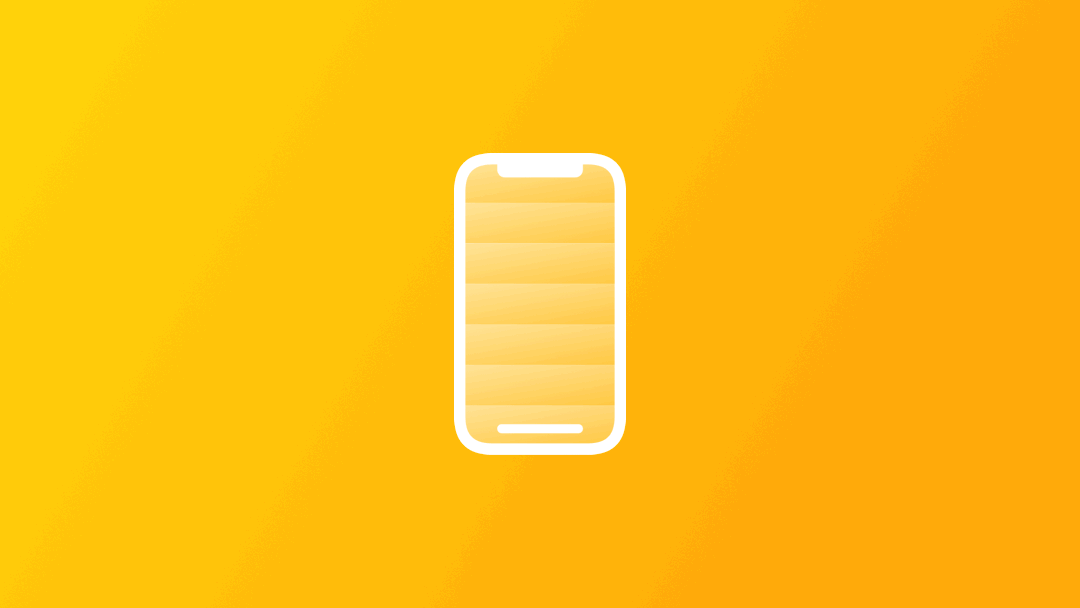