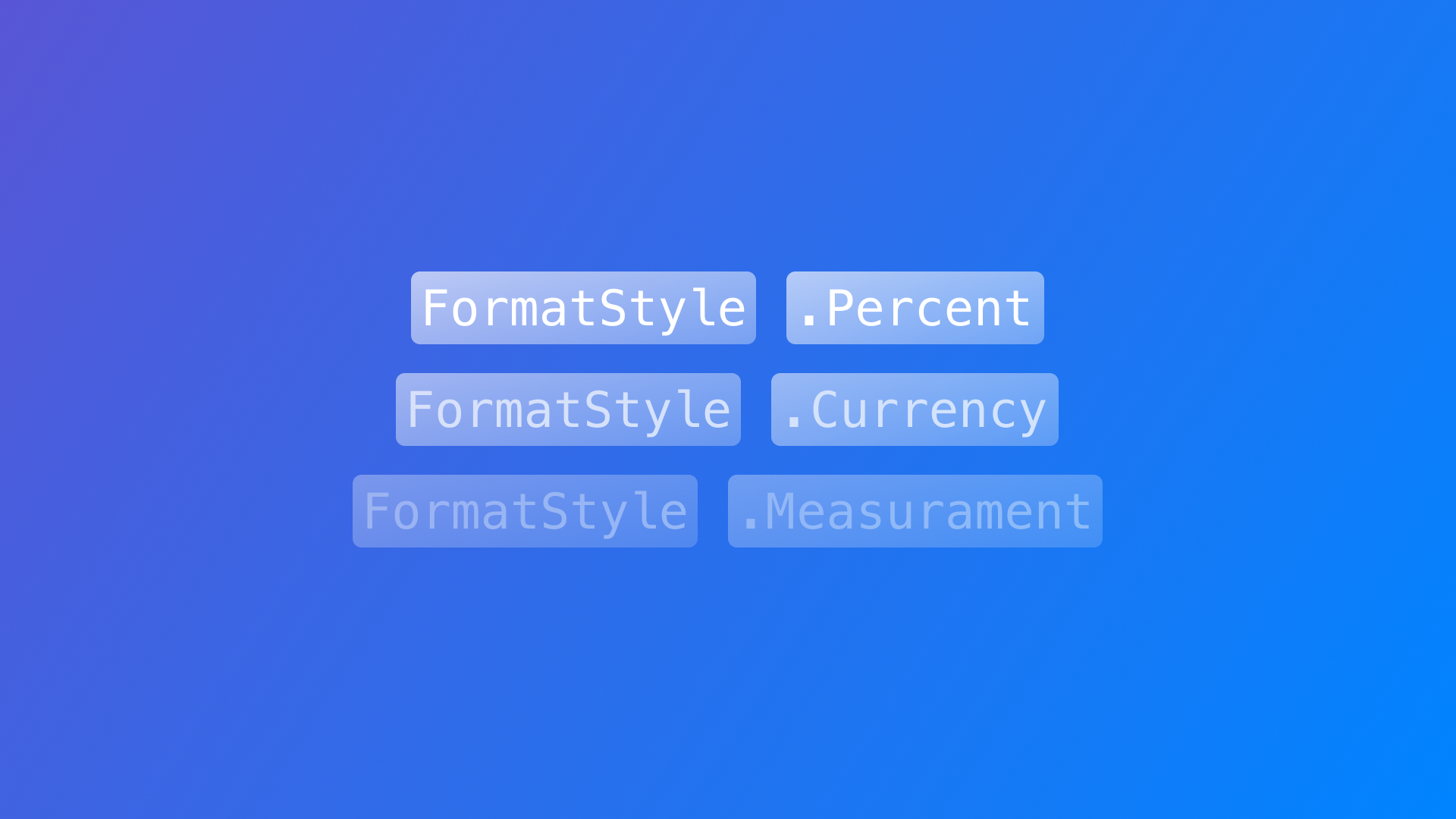
Formatting data as text in a Text view in SwiftUI
Learn how to format different types of data within the Text view in SwiftUI
SwiftUI’s Text
view offers a wide range of options for formatting text, allowing us to display currencies, dates, measurements, and other types of data in a user-friendly manner.
The Text(_:format:)
initialiser allows us to specify a particular formatting method ensuring that our data is presented correctly.
In this reference, we will delve into various formatting techniques showcasing how to use different text formatters in SwiftUI.
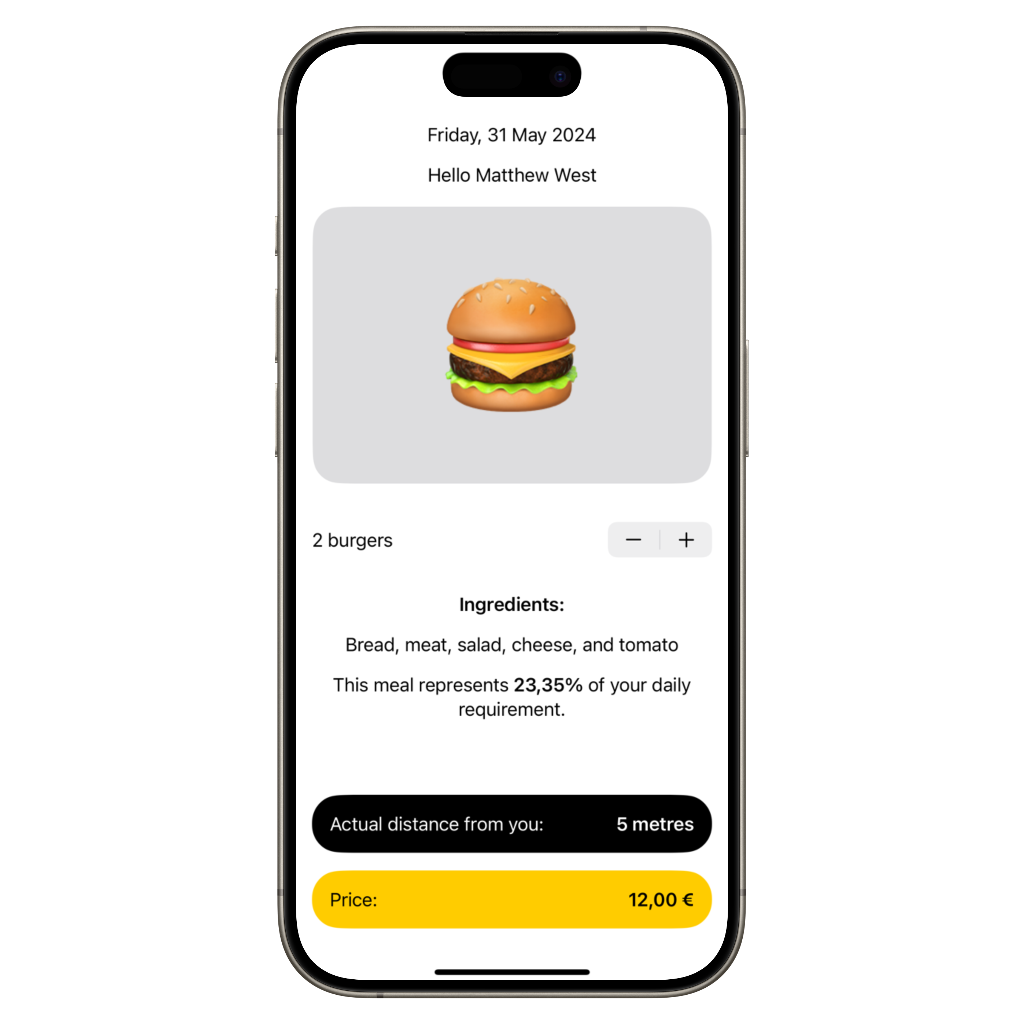
Currency formatting
When dealing with displaying currency as text you can use the Decimal.FormatStyle.Currency
formatter.
It allows you to display numbers as currency, complete with the appropriate symbols and decimal places. As an example, applying the currency style to the number 1000 would yield "$1,000.00".
struct ContentView: View {
var body: some View {
// Output: 72,30 £
Text(72.3, format: .currency(code: "GBP"))
}
}
You can get the currency code of the user system settings so you can use it when formatting the text.
let systemCurrency = Locale.current.currency?.identifier ?? "EUR"
Text(72.3, format: .currency(code: systemCurrency))
Percentage formatting
When dealing with displaying a percentage value as text you can use the Decimal.FormatStyle.Percent
formatter. It will quickly convert a decimal number to its percentage equivalent.
struct ContentView: View {
var decimal : Double {
23.4 / 100
}
var body: some View {
// Output: 23,4%
Text(decimal, format: .percent)
}
}
Measurement formatting
When dealing with measurements in SwiftUI you can use the Measurement.FormatStyle
formatter. It formats a Measurement
object representing quantities and units of measure.
Displaying a Measurement
object within a Text
view allows you to properly format how the measurement is shown by using the formatter object.
This ensures that measurements are presented accurately and properly for different regions and user preferences.
struct ContentView: View {
let length = Measurement(value: 5.0, unit: UnitLength.feet)
var body: some View {
// Output: 1,5 metres
Text(length, format: .measurement(width: .wide))
}
}
Date formatting
When dealing with date and time in SwiftUI you can use the Date.FormatStyle
formatter. It helps you displaying date and time values as text in a in a variety of styles, based on your needs.
struct ContentView: View {
@State private var myDate = Date()
var body: some View {
// Output: 7 Jun 2024 at 15:00
Text(myDate, format: Date.FormatStyle(date: .abbreviated, time: .shortened))
}
}
List formatting
When dealing with a list of elements in SwiftUI and you need to represent them as text you can use the ListFormatStyle
formatter. It converts an array into a single line of text that uses commas and conjunctions based on the current locale.
struct ContentView: View {
let items: [Int] = [100, 1000, 10000, 100000, 1000000]
var body: some View {
Text(items, format: .list(memberStyle: .number, type: .and))
}
}
Person name formatting
In Swift you can represent the information of a person name using the PersonNameComponents
type. This can be useful when working with user profile information or when displaying and formatting names in your app.
When displaying the person name on the interface you can use the PersonNameComponents.FormatStyle
formatter to define the proper way of presenting it in that context.
struct ContentView: View {
let name = PersonNameComponents(namePrefix: "Dr.",
givenName: "Thomas",
middleName: "Louis",
familyName: "Clark",
nameSuffix: "Jr.",
nickname: "Tom")
var body: some View {
Text(name, format: .name(style: .medium))
}
}
Applying inflection
The inflection
parameter is used to automatically handle pluralization based on a dynamic value, like a number that represents quantity. It's particularly useful when you want to display text that needs to represent properly a accompanying number representing quantity.
struct ContentView: View {
@State var count: Int = 0
var body: some View {
Text("^[\\(count) company](inflect: true)")
.onTapGesture {
count += 1
}
}
}
There is much more!
We saw how powerful the Text
view can be when it comes to properly displaying different formats of content, ensuring that your app's information is presented in a dynamic and contextually appropriate manner.
There are many other formats made available through the Swift standard library on Foundation. It is worth exploring it even further, you might find something there that can make your life much easier when formatting the data to be presented on your applications user interface.
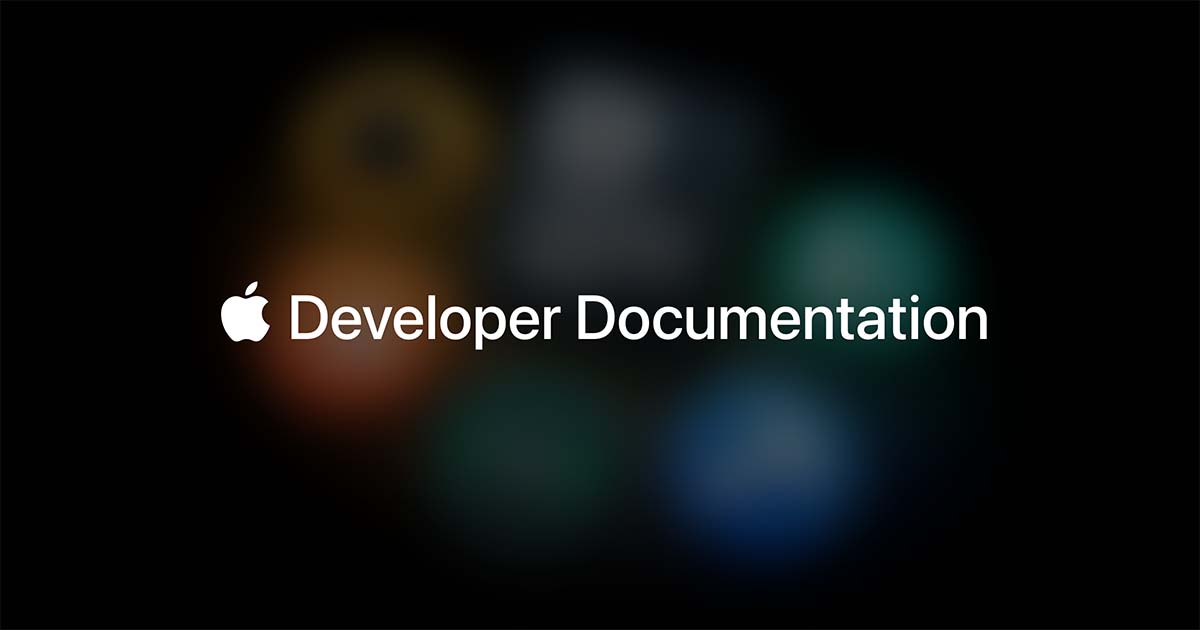
Take a look at the Data Formatting page in the official Apple Developer Documentation to find out more.