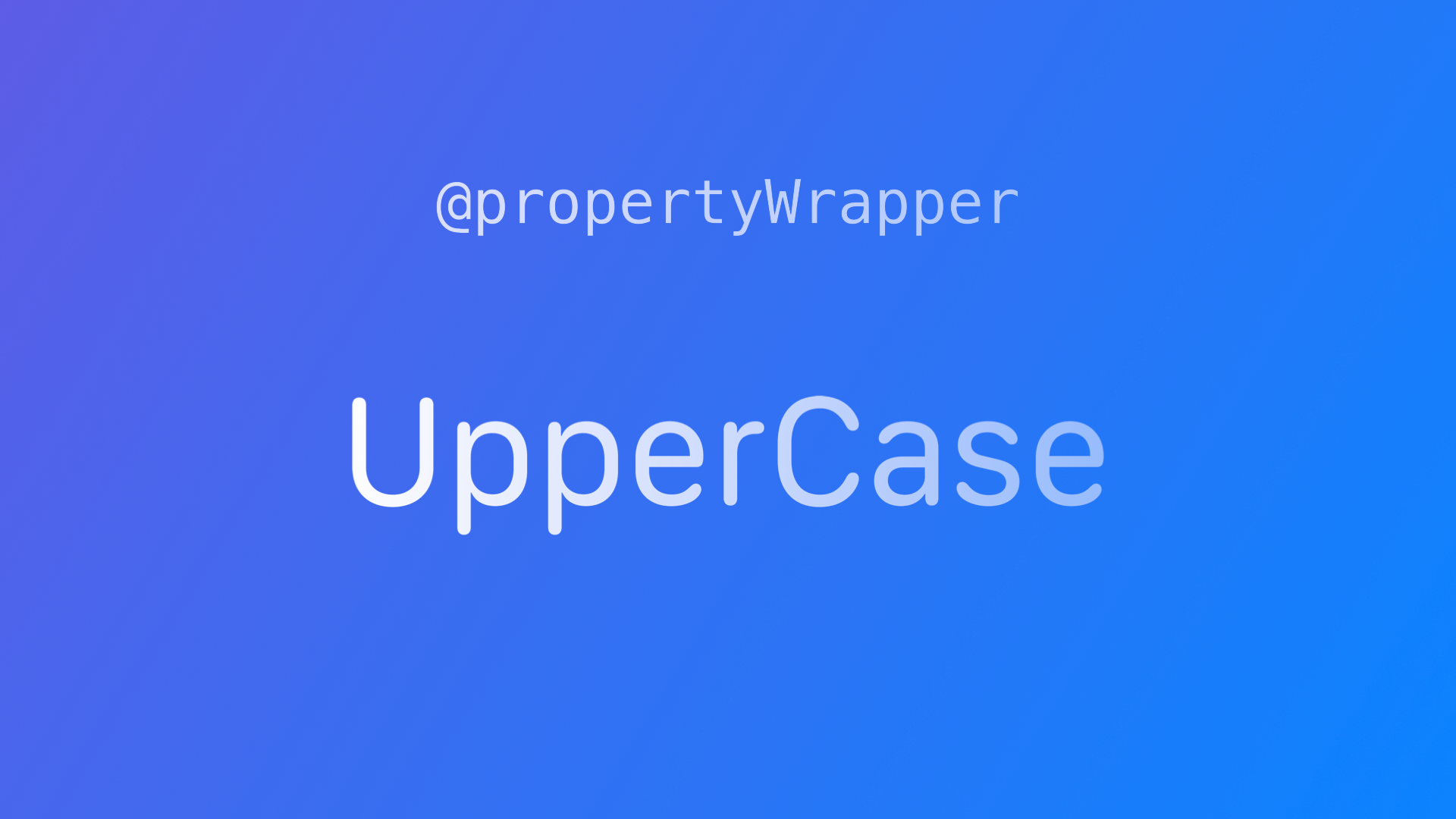
@propertyWrapper: UpperCase String
With this @propertyWrapper code snippet you will be able to wrap String values into wrapped values of only upper case characters.
Property wrappers serve as a new type to wrap properties and add additional logic if needed. It's part of Swift since version 5.1 and can be useful to validate values with a set of rules or tranform values to match certain requirements etc.
As a simple example, you might want to ensure that String is using upper case, for example to correctly describe the abbreviation of federal states in the U.S. like California (CA) or New York (NY) or flight numbers and airport short codes such as Lufthase Flight 456 (LH456) and Frankfurt International Airport (FRA).
The property wrapper can be defined as a class with a private(set) property value that returns a wrappedValue by transorming the internal value to an uppercase String with the .uppercased() method.
@propertyWrapper
class UpperCase {
private(set) var value: String = ""
var wrappedValue: String {
get { value }
set {
value = newValue.uppercased()
}
}
}
struct Flight {
@UpperCase
var number: String
@UpperCase
var destination: String
init(number: String) {
self.number = number
}
}
var flight = Flight(number: "lh456")
flight.destination = "fra"
print("\(flight.number) to \(flight.destination)")
//LH456 to FRA
There are many other cool things you can do with @porpertyWrapper. For more conclusive coverage, consider "Property Wrappers in Swift explained with code examples" by Antoine van der Lee or "Property wrappers in Swift" by John Sundell. Both provide an excellent overview.