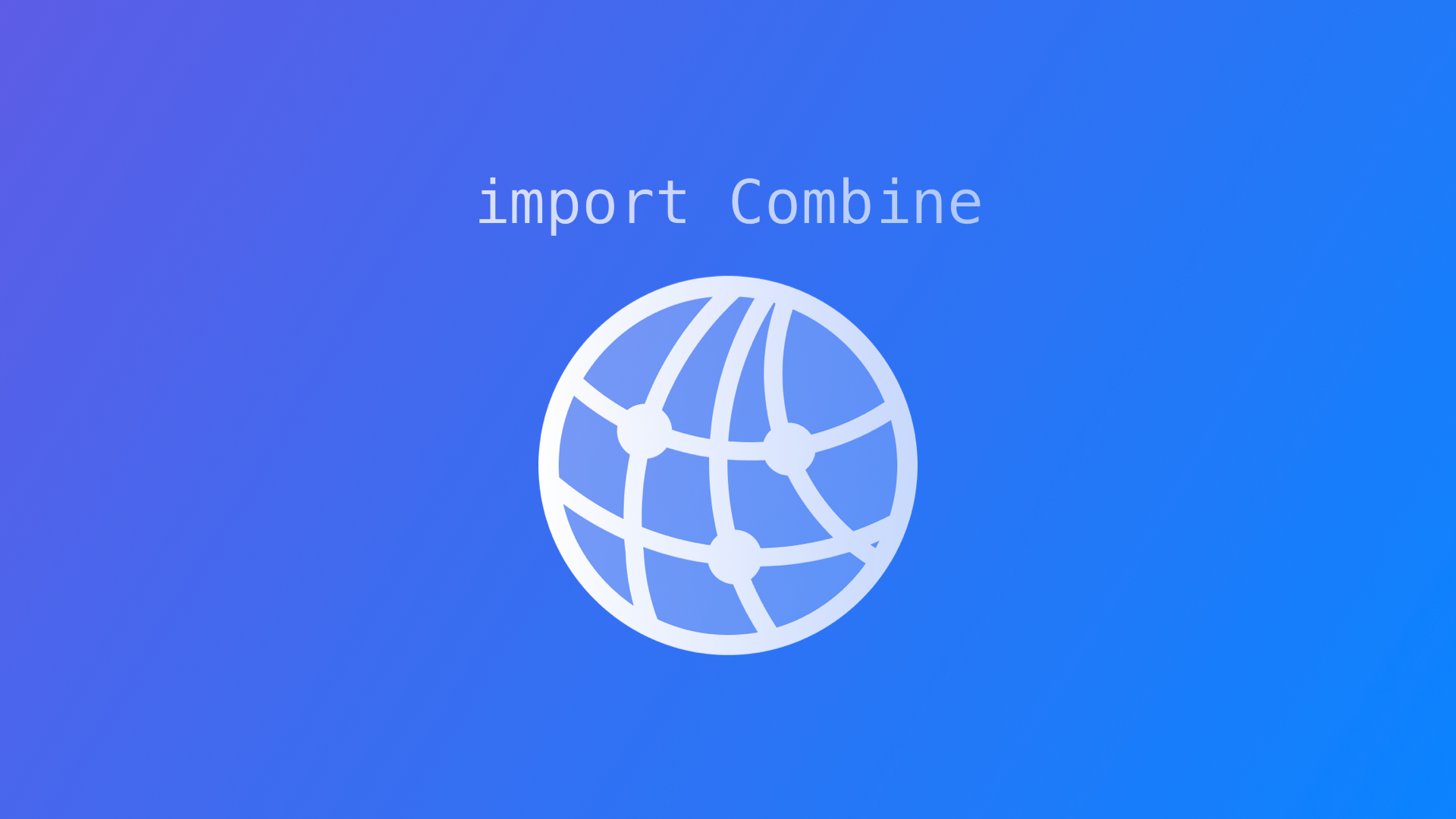
Combine: Networking and the dataTaskPublisher
With this code snippet you will be able use the new dataTaskPublisher in URLSession to handle asynchronous download of data.
This brief overview will demonstrate some basic features that may come in handy when working with publishers in Combine, Apple's framework to handle asynchronous events by combining event-processing operators. The Publisher protocol declares a type that transmits a sequence of values over time that subscribers can receive as input by adopting the Subscriber protocol.
A typical use case for Combine would be handling asynchronous download of data from the internet using a URLSession dataTask. This can be achieved by using the new URLSession.shared.dataTaskPublisher(for: URL)
. Let's have a look.
For example:
import Foundation
import Combine
struct Post: Codable {
let title: String
let body: String
}
func getPosts() -> AnyPublisher<[Post], Error> {
guard let url = URL(string: "https://jsonplaceholder.typicode.com/posts") else {
fatalError("Invalid URL")
}
return URLSession.shared.dataTaskPublisher(for: url).map { $0.data }
.decode(type: [Post].self, decoder: JSONDecoder())
.receive(on: RunLoop.main)
.eraseToAnyPublisher()
}
let cancellable = getPosts().sink(receiveCompletion: { _ in }, receiveValue: { print($0) })
c
The Post
struct defines a simple data stucture that can be used with a JSONDecoder()
to decode the boilerplate JSON data received from https://jsonplaceholder.typicode.com/posts. The dataTaskPublisher
receives that data from the url, maps the results to access the data before decoing it and than wraps the publisher into an AnyPublisher
. The cancellable sinks to the publisher by creating the subscriber and requesting values.
The values received from the publisher are then printed to the console. Of course, this is where you would do with the values whatever you intend to do. All in all, it is a quite simple procedure.
Where to go next?
If you are interested into knowing more about working with Combine and the various operators available to shape the sequence of values send by publishers and received by subscribers, check our other articles on Combine that will be released over the next days and weeks.
They will cover how to work with filters, sequence and transformation operatators or timers, how to use the dataTaskPublisher, how to combine publishers as well as how to debug Combine messages and much more.
To be notifed when new material is out, join our free mailing list.