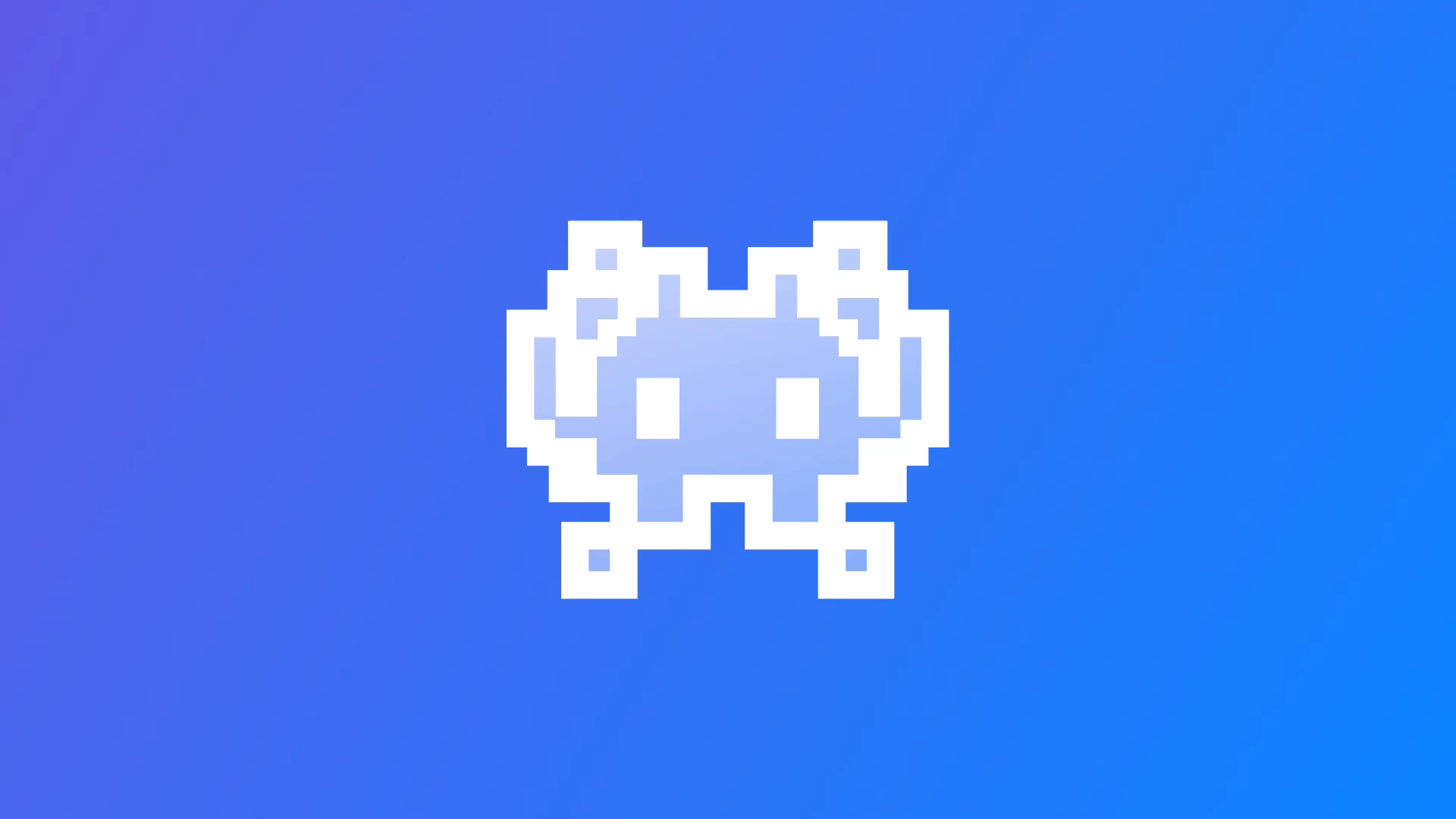
Sprite Animation with SpriteKit
This reference article covers how to create animations using SpriteKit SKSpriteNode and textures.
When creating a game using SpriteKit one of the first things we want to do is guarantee that our game feels alive. To bring that touch of life into the game a common practice is to animate the game elements.
How to create sprite animations in a SpriteKit game? Let's have a look.
The first thing you need to do is create a Sprite Atlas in your Assets folder. The Sprite Atlas is a special type of folder that will collect images that are used together often to improve memory usage and rendering performance.
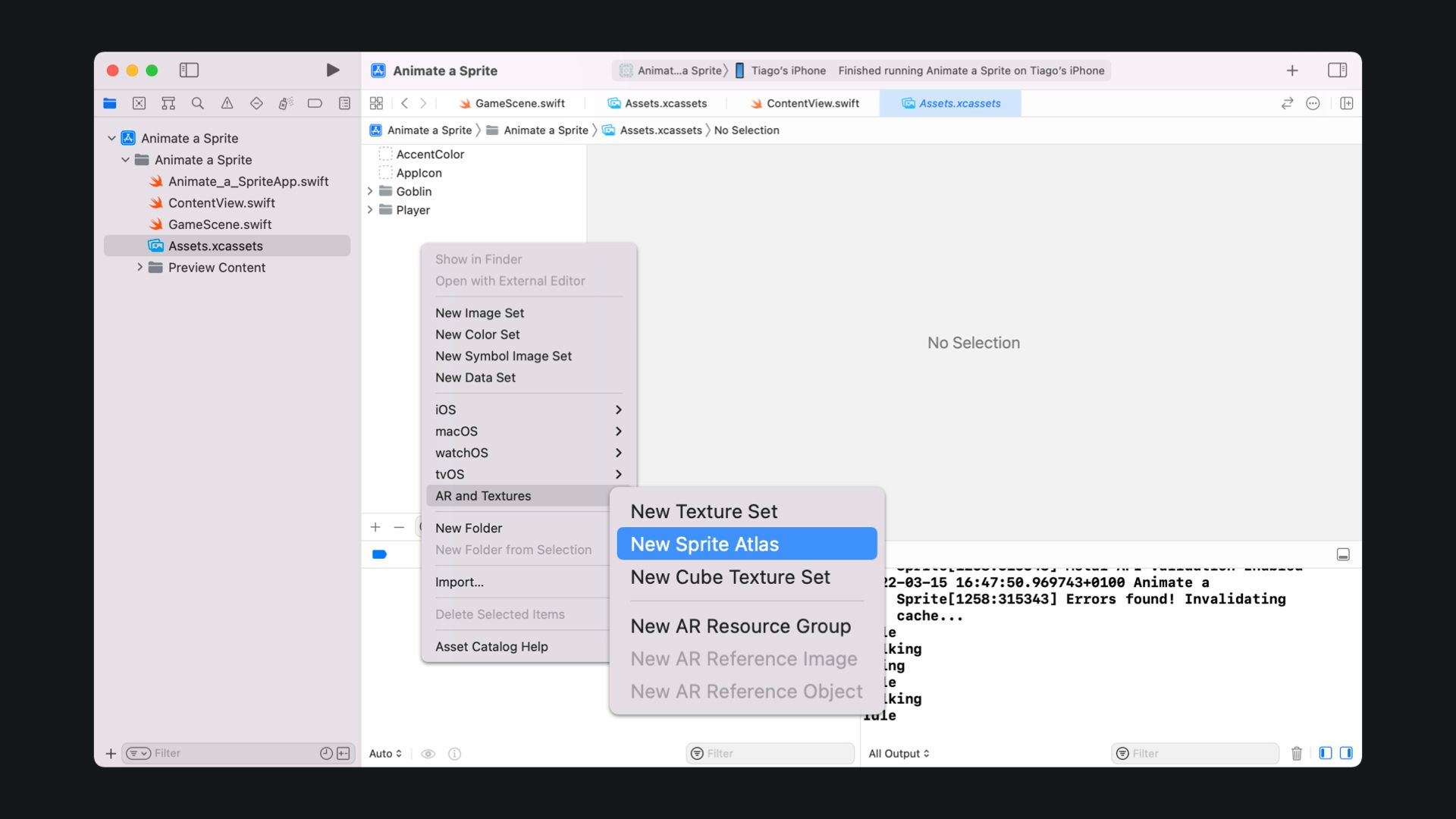
Once the Sprite Atlas is created, place the sprites for your animation inside.
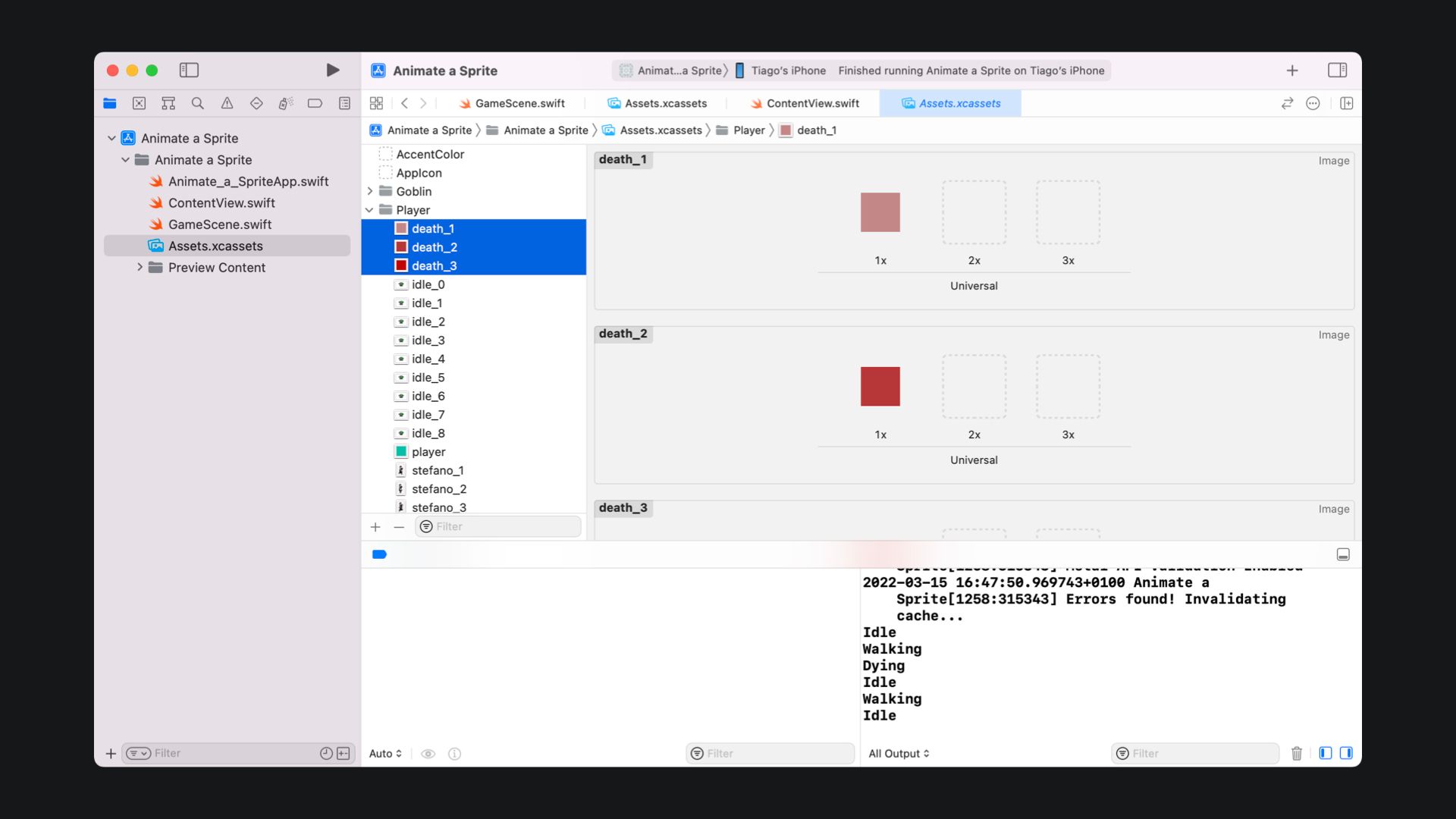
To know more about Sprite Atlases you can check the article About Texture Atlases on the official Apple documentation portal.
About Texture Atlases - Article at the Apple Developer Portal
You are now ready to use those images in your SpriteKit based game.
To easily access your Sprite Atlas create a computed property return a SKTextureAtlas
, like in the example below. Make sure that the value of the name parameter is the same as the Sprite Atlas you created previously.
private var playerAtlas: SKTextureAtlas {
return SKTextureAtlas(named: "Player")
}
Accessing a Sprite Atlas on code
Now let’s load the textures to be used in our animation cycles using our Sprite Atlas. Load the textures in the order of your animation cycle.
private var playerIdleTextures: [SKTexture] {
return [
playerAtlas.textureNamed("idle_1"),
playerAtlas.textureNamed("idle_2"),
playerAtlas.textureNamed("idle_3"),
playerAtlas.textureNamed("idle_2")
]
}
Loading textures to be used in an animation
You can also preload your textures, for better performance. To know more about texture performance read the article Maximizing Texture Performance at the official Apple documentation portal.
Maximizing Texture Performance - Article at the Apple Developer Portal
func startIdleAnimation() {
let idleAnimation = SKAction.animate(with: playerIdleTextures, timePerFrame: 0.3)
player.run(SKAction.repeatForever(idleAnimation), withKey: "playerIdleAnimation")
}
Animating a SKSpriteNode
This is what your game scene might look like.
class GameScene: SKScene {
var player: SKSpriteNode!
private var playerAtlas: SKTextureAtlas {
return SKTextureAtlas(named: "Player")
}
private var playerTexture: SKTexture {
return playerAtlas.textureNamed("player")
}
private var playerIdleTextures: [SKTexture] {
return [
playerAtlas.textureNamed("idle_0"),
playerAtlas.textureNamed("idle_1"),
playerAtlas.textureNamed("idle_2"),
playerAtlas.textureNamed("idle_3"),
playerAtlas.textureNamed("idle_4"),
playerAtlas.textureNamed("idle_5"),
playerAtlas.textureNamed("idle_6"),
playerAtlas.textureNamed("idle_7"),
playerAtlas.textureNamed("idle_8")
]
}
private func setupPlayer() {
player = SKSpriteNode(texture: playerTexture, size: CGSize(width: 70, height: 46))
player.position = CGPoint(x: frame.width/2, y: frame.height/2)
addChild(player)
}
override func didMove(to view: SKView) {
backgroundColor = SKColor.white
self.setupPlayer()
self.startIdleAnimation()
}
func startIdleAnimation() {
let idleAnimation = SKAction.animate(with: playerIdleTextures, timePerFrame: 0.05)
player.run(SKAction.repeatForever(idleAnimation), withKey: "playerIdleAnimation")
}
}
Example of a game scene running a sprite animation
Wrapping up
Now that you know how to run animation using sprite atlas, textures and a SKSpriteNode
start thinking about how would you organize your code if your nodes have different states.
What if your player has a death animation, an idle animation, and a walking animation? How would you change between those animations?
We are going to address this and much more in future reference articles and tutorials.