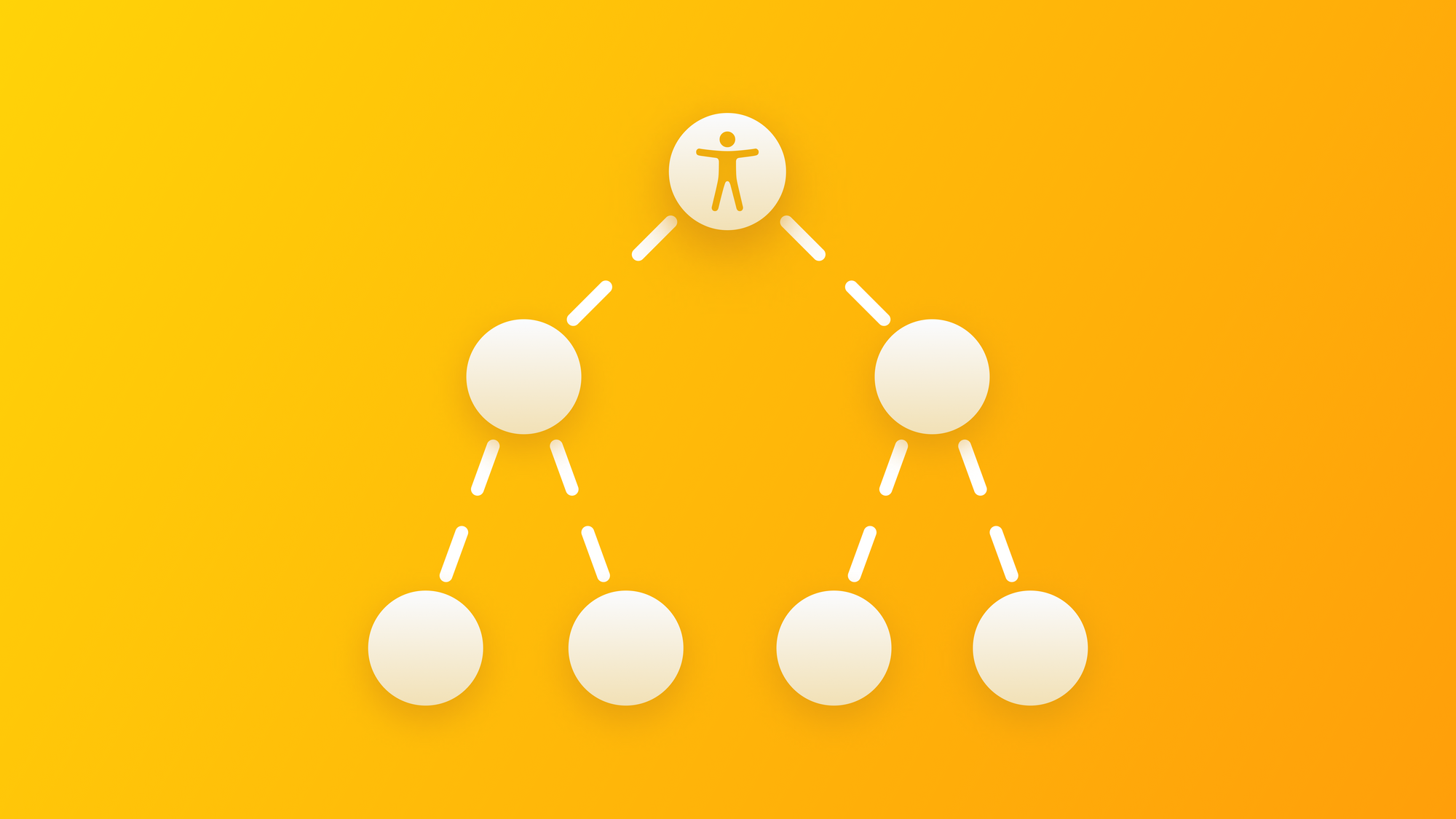
Understanding the Accessible User Interface
Understand the Accessible User Interface on applications created with UIKit and SwiftUI.
As developers, we often focus on creating appealing and user-friendly user interfaces, carefully organizing the variety of components that we have available and crafting new ones. However, our responsibility goes beyond creating traditional user interfaces, we must also delve into the domain of accessibility.
Apple's operating systems create a bridge for users to interact with our applications through assistive technologies such as VoiceOver, Voice Control and Switch Control. They in fact create another type of interface with our application: the Accessible User Interface (AUI in short), also referred to as the Accessibility Tree.
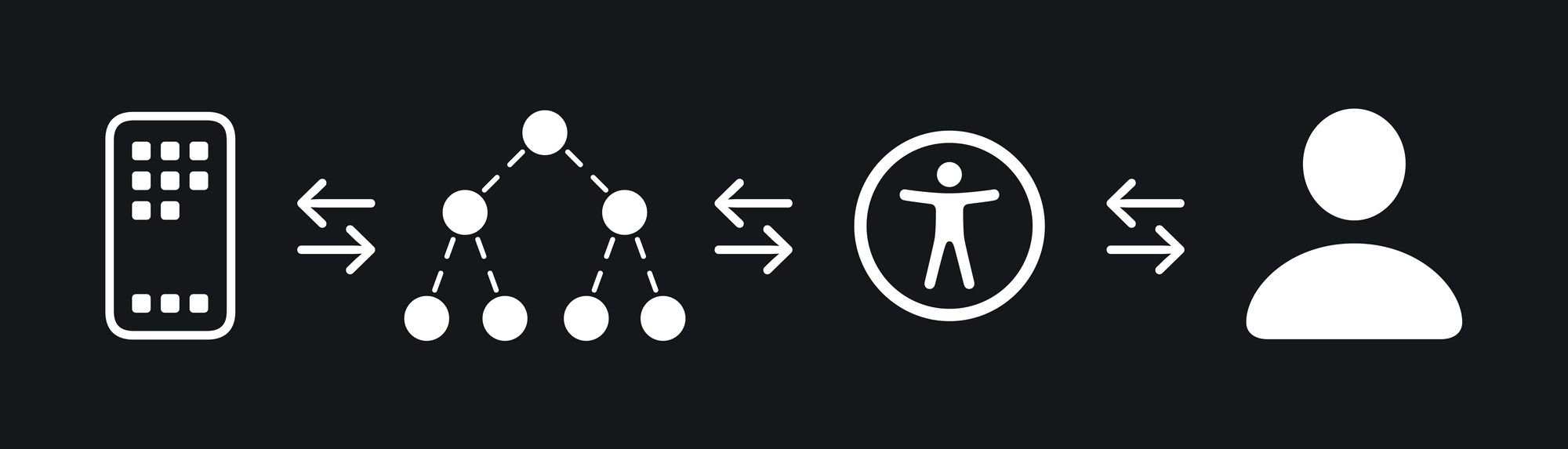
The differences between the UI and AUI
The Accessible User Interface (AUI) and the standard User Interface (UI) often diverge. Notably, their sorting priorities can differ, affecting the hierarchy we usually establish using font styles and weights. However, this hierarchy isn't always conveyed seamlessly through assistive technologies, which present elements in their natural reading language and original sorting order.
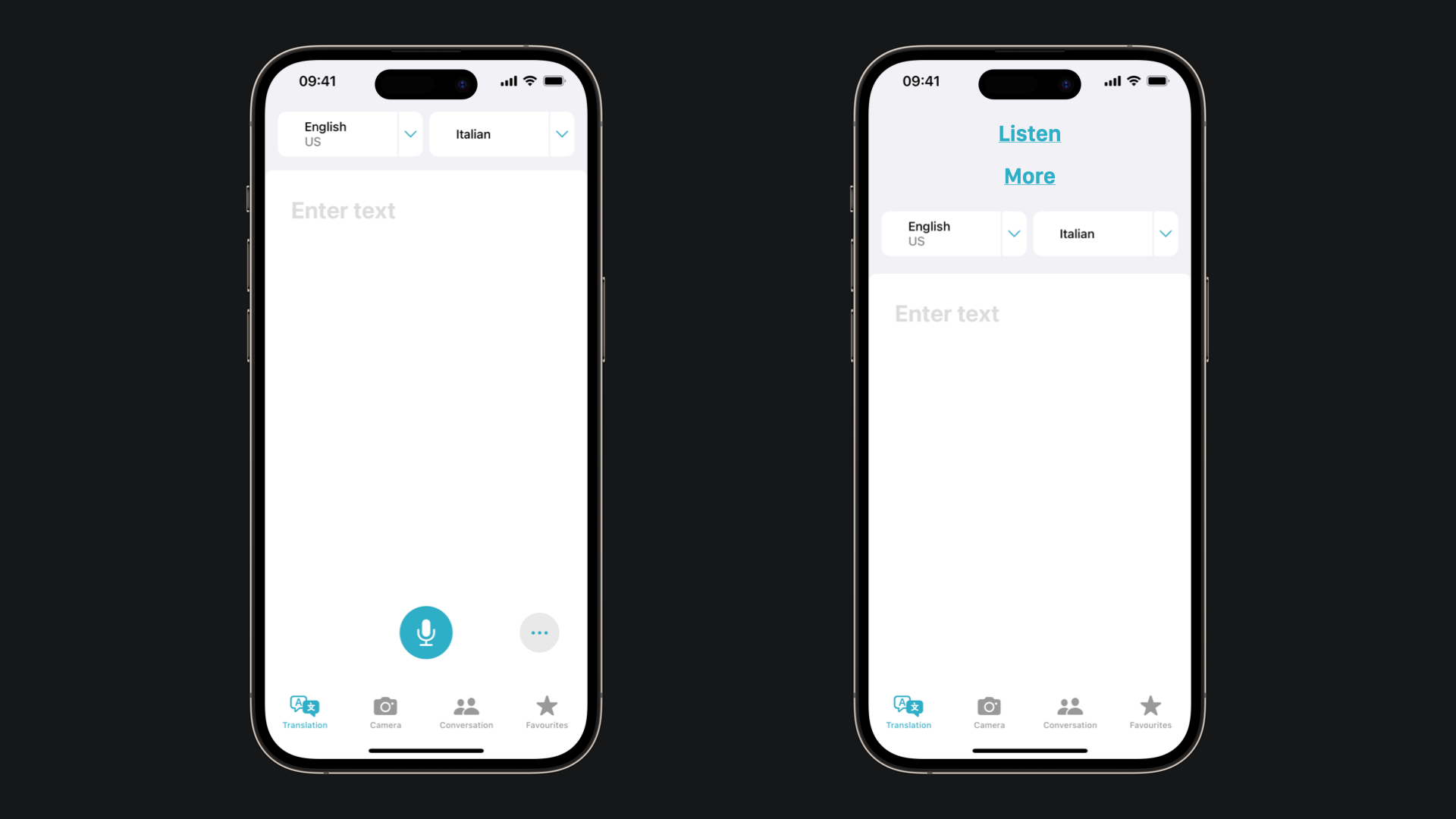
This can create a mismatch between perceived visual hierarchy and its translation via assistive tools. Another example is text cues that may not be as helpful for users relying on assistive technologies as they are for sighted users, the AUI enriches user experiences by providing additional information, including important hints for interactive elements. On the other hand, certain visual elements that are significant in UI may be omitted from AUI, like decorative or non-interactive components, that are important for visual aesthetics but not for conveying functional information.
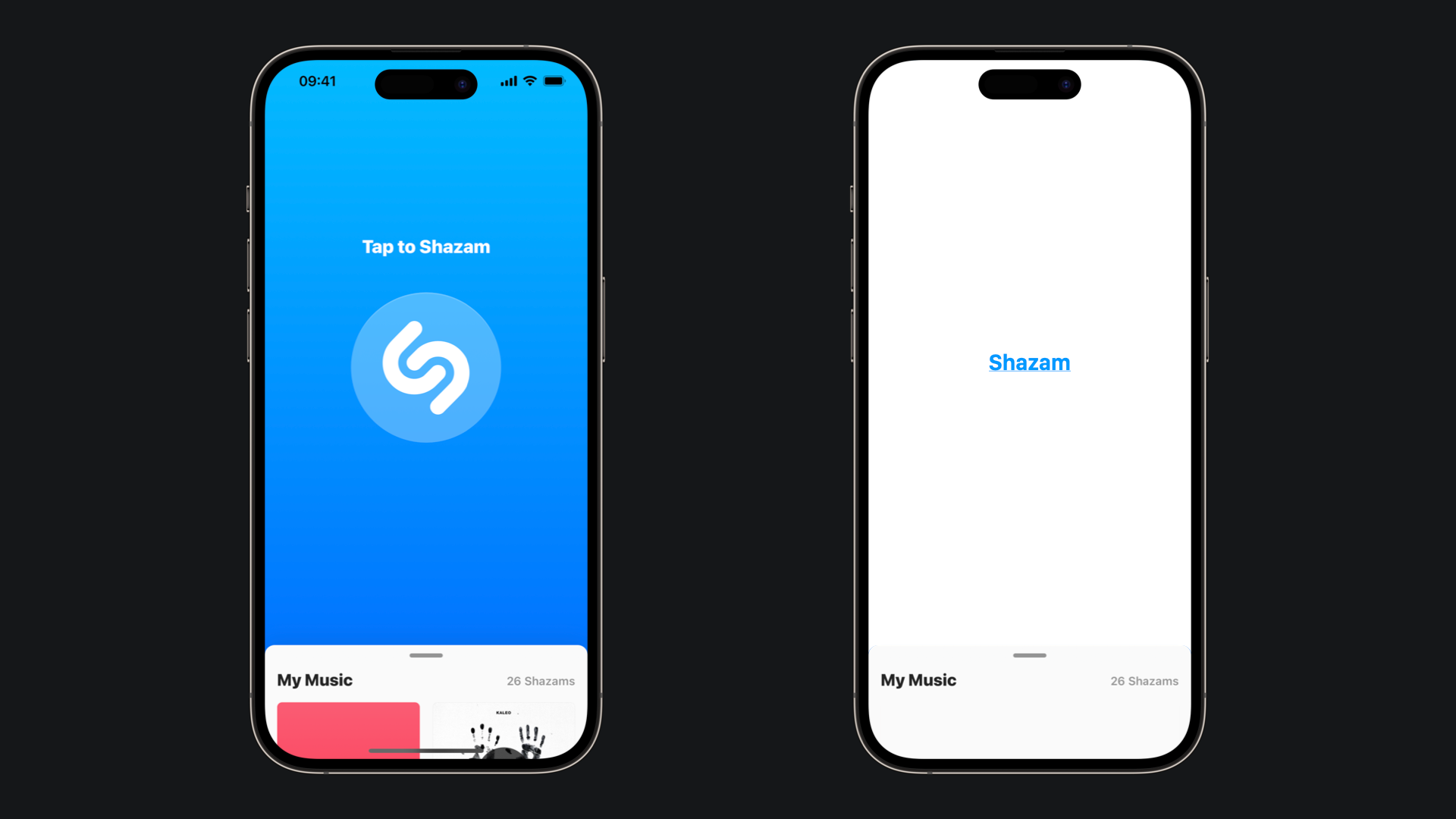
How the AUI is created
Both UIKit and SwifUI support basic accessibility information for system-provided components. Typically, the standard accessibility features are readily available without any extra effort on our part. However, as developers, we have the opportunity to enhance the app's accessibility experience by focusing on the more refined details, going beyond the standards and making it a great experience.
Let's have a look.