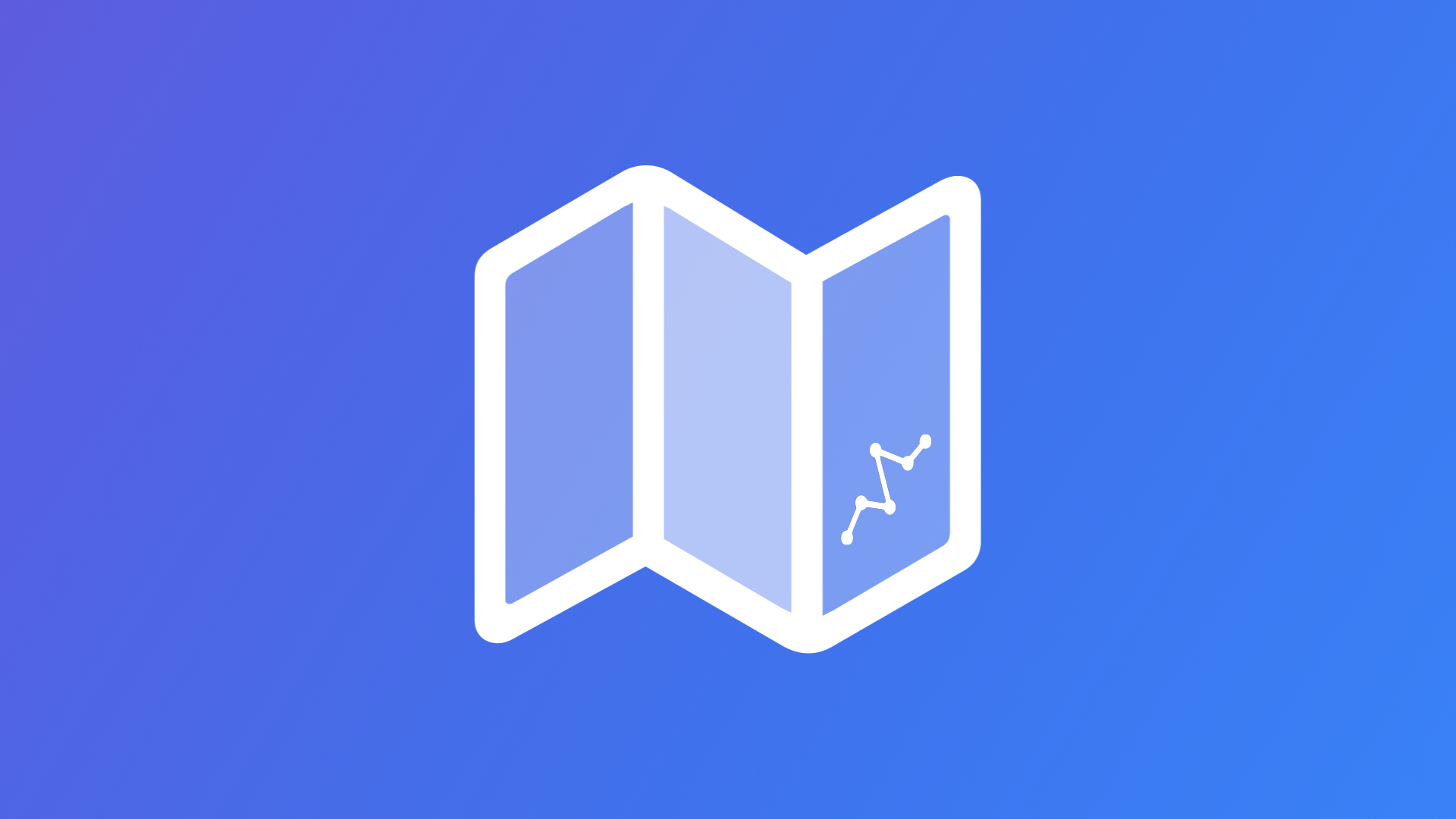
Using MapPolyline overlays in MapKit with SwiftUI
Learn how to draw a line on a Map on a SwiftUI application using MapPolyline.
A MapPolyline
is a map content that when placed inside a Map
view draws an open polygon overlay consisting of one or more connected line segments. It can be drawn based on any number of coordinates, MKMapPoint
, a MKRoute
or a MKPolyline
.
import SwiftUI
import MapKit
struct LineOnMapView: View {
/// The provided walking route
let walkingRoute: [CLLocationCoordinate2D] = [
CLLocationCoordinate2D(latitude: 40.836456,longitude: 14.307014),
CLLocationCoordinate2D(latitude: 40.835654,longitude: 14.304346),
CLLocationCoordinate2D(latitude: 40.836478,longitude: 14.302593),
CLLocationCoordinate2D(latitude: 40.836936,longitude: 14.302464)
]
var body: some View {
Map {
/// The Map Polyline map content object
MapPolyline(coordinates: walkingRoute)
.stroke(.blue, lineWidth: 5)
}
}
}
When providing the sequence of coordinates it is important to ensure that they are in the correct order, because the MapPolyline
will be rendered precisely in the order in which the points are presented.
MapPolyline
conforms to the MapContent
protocol, which is used to construct map elements such as controls, markers, annotations, and other shape overlays.
The relevant modifiers are:
.stroke(_:lineWidth:)
: defines the width and color of the line.strokeStyle(style:)
: defines how the line is drawn using aStrokeStyle
object. Used to make dashed lines, for example.mapOverlayLevel(level:)
: defines which elements of the map the line will be drawn on top of
With a stroke style, you can have a dashed line on your map and you can even color the stroke with a gradient, like in the example below.
let strokeStyle = StrokeStyle(
lineWidth: 3,
lineCap: .round,
lineJoin: .round,
dash: [5, 5]
)
let gradient = Gradient(colors: [.red, .green, .blue])
var body: some View {
Map {
MapPolyline(coordinates: walkingRoute)
.stroke(gradient, style: strokeStyle)
}
}