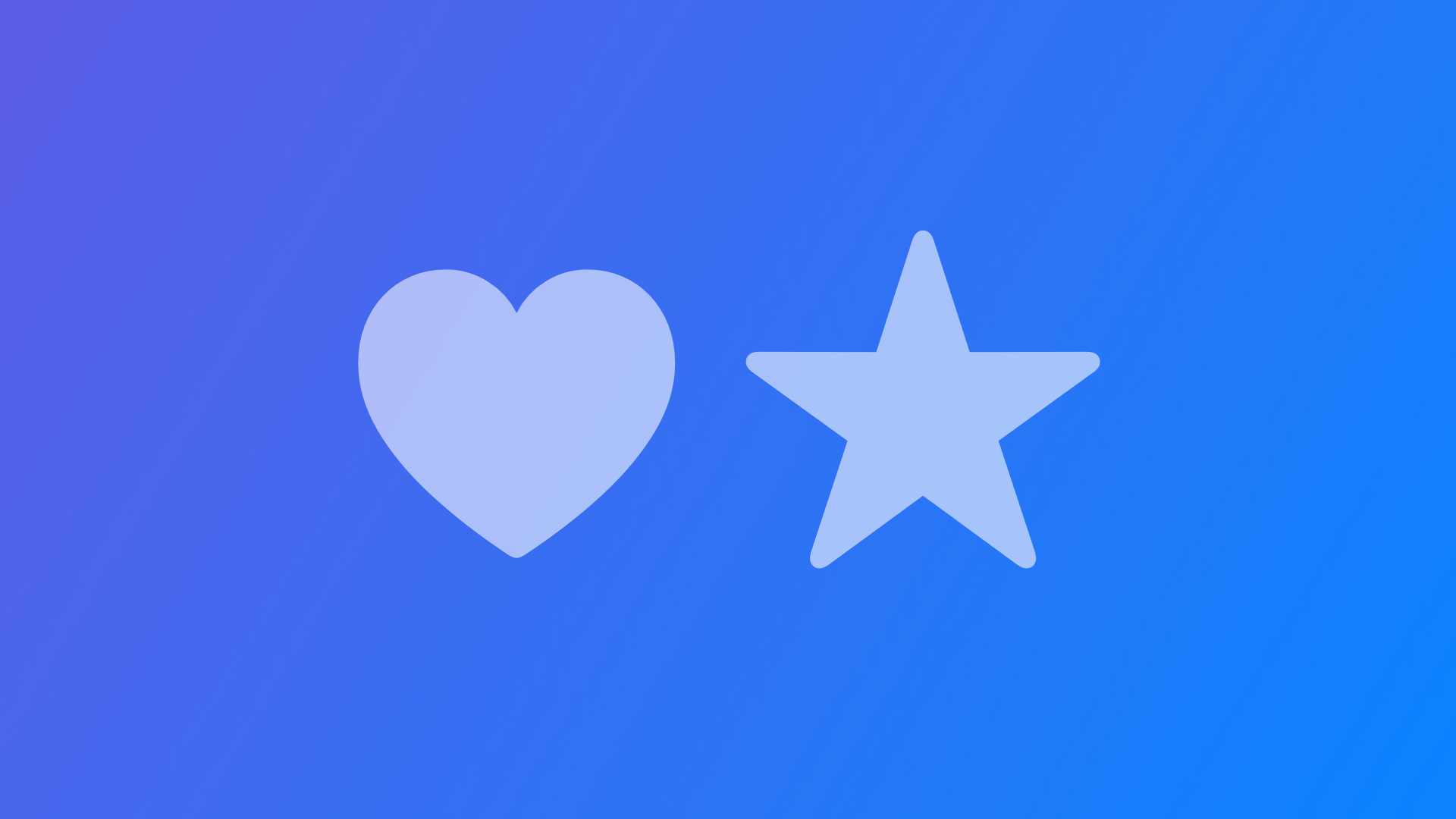
Using SF Symbols in SwiftUI
This reference article covers how to use SF Symbols in SwiftUI and the most common modifiers associated with them.
SF Symbols is a library of icons designed to be used with the San Francisco font, the system font for Apple platforms. You can have access to the library of over 3,000 symbols by downloading the mac app available on the official Apple Website.
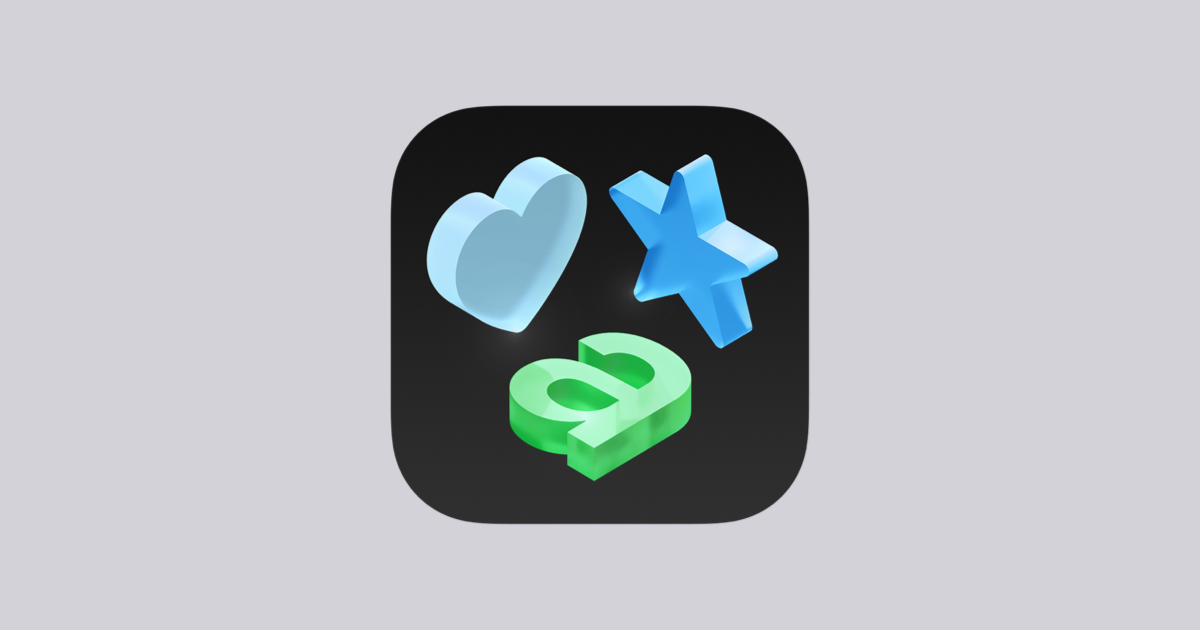
And for guidelines on how to use them on your applications check the Human Interface Guidelines page dedicated to them. In there you can see all the different properties you can change and even how to create your custom symbols.

Another big plus is that using those icons in your interfaces made with SwiftUI requires only creating a new image using the proper name.
Image(systemName: "house")
You can change multiple properties of the SF Symbols you use on your interface by using the number of different modifiers available. Let's have a look.
Color
To change the color of an SF Symbol we can use the modifiers .foregroundColor()
and .foregroundStyle()
.
By changing the foreground color you will paint the symbol with a single color.
Image(systemName: "bell.badge")
.foregroundColor(.purple)
Changing the color of the symbol
Using by applying foreground styles to the symbol you can take advantage of the fact that some symbols support multiple colors, like in the example below.
Image(systemName: "bell.badge")
.foregroundStyle(.purple, .gray)
Adding styles to the symbol
Rendering Mode
SF Symbols also have multiple rendering modes allowing you to decide how the icon will be colored. To set up the rendering mode of the symbol use the modifier .symbolRenderingMode()
.
Image(systemName: "bell.badge")
.symbolRenderingMode(.monochrome)
.foregroundStyle(.purple)
Image(systemName: "theatermasks")
.symbolRenderingMode(.hierarchical)
.foregroundStyle(.purple)
Image(systemName: "theatermasks")
.symbolRenderingMode(.palette)
.foregroundStyle(.purple, .gray)
Image(systemName: "bell.badge")
.symbolRenderingMode(.multicolor)
.foregroundStyle(.purple)
Examples of different rendering modes. Check the Human Interface Guidelines for more information on how each rendering mode affects an SF Symbol.
For an explanation of each rendering, mode checks the documentation page for the SymbolRenderingMode
structure.
Documentation page for the SymbolRenderingMode structure.
Keep in mind that when working with the palette rendering mode, the symbol will be rendered as multiple layers, because of that you can apply different styles to each layer with the .foregroundStyle()
modifier.
Image(systemName: "battery.100.bolt")
.symbolRenderingMode(.palette)
.foregroundStyle(.green, .tertiary, .primary)
Weights and Scale
You can treat SF Symbols as a font or as an image, allowing you to modify their size in multiple ways.
With the .font()
modifier you can match the symbol to your text style using the default
Image(systemName: "house")
.font(.title)
Image(systemName: "house")
.font(.headline)
Image(systemName: "house")
.font(.caption)
You can also set the size and the weight of the symbol by providing a system font with a custom size and weight to the .font()
modifier.
Image(systemName: "house")
.font(.system(size: 24.0, weight: .black))
Another way to customize the size of a symbol is using the .imageScale()
modifier.
Image(systemName: "house")
.imageScale(.small)
Image(systemName: "house")
.imageScale(.medium)
Image(systemName: "house")
.imageScale(.large)
Variants
There are several different design variants that you can apply to an SF Symbol. You can add a variant to a symbol by using the .symbolVariant()
modifier.
Image(systemName: "square")
.symbolVariant(.slash)
.symbolVariant(.fill)
// The order doesn't matter if the symbol supports the variants
Image(systemName: "square")
.symbolVariant(.fill)
.symbolVariant(.slash)
You can also apply variants to a group of symbols and if one or more of the symbols don’t support a specific variant it will just be ignored.
VStack {
Image(systemName: "checkmark")
Image(systemName: "person")
}
.symbolVariant(.fill)
.symbolVariant(.circle)
You can check all the available variants in the documentation for symbol variants in SwiftUI.
Documentation page for the SymbolVariants structure