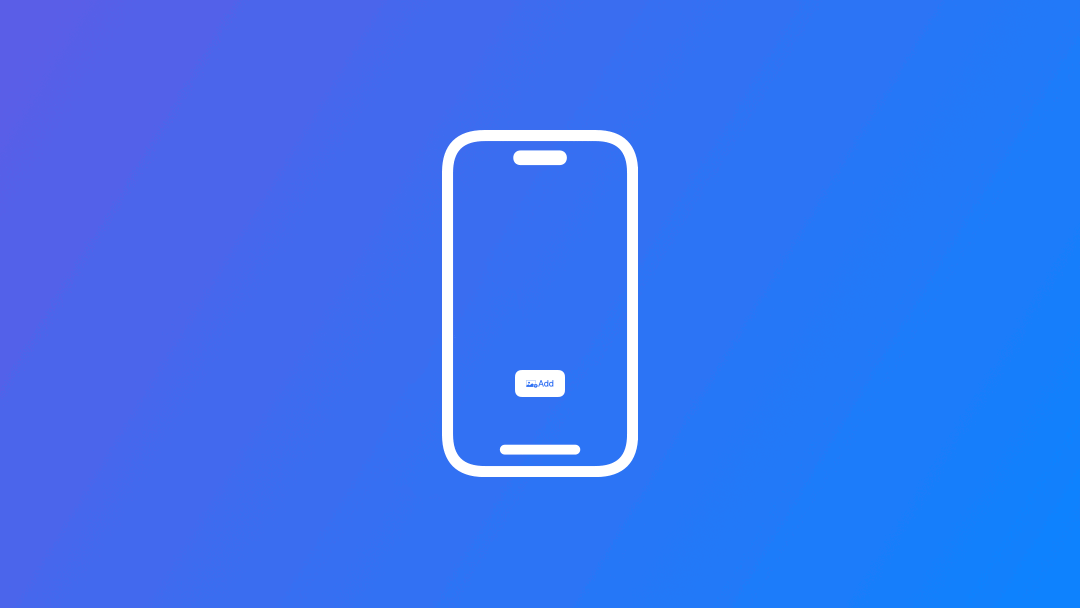
Picking an Image from the Photos Library in a SwiftUI App
Learn how to use the photos picker to allow users to select a photo from their photo library.
The PhotosPicker
component enables users to import photos or videos from their photos library directly within a SwiftUI app. This reference will guide you through the implementation of this component.
To work with image and video assets managed by the Photos app, we must first import the PhotosUI
framework. Next, we create a new variable of PhotosPickerItem
type to store the selected image.
After that we can use the PhotosPicker
component inside our View
.
The PhotosPicker
initializer needs a label to describe the action of choosing an item from the photo library and a variable that for the selection parameter. Additionally, we can also specify what type of media we are looking for.
import SwiftUI
import PhotosUI
struct ContentView: View {
@State private var avatarPhotoItem: PhotosPickerItem?
var body: some View {
VStack {
PhotosPicker("Select image",
selection: $avatarPhotoItem,
matching: .images)
}
}
}
Using the PhotosPicker component on a SwiftUI View
To show the selected image on your interface you need to create a representation of the picked file as a SwiftUI type. You do it by using the loadTransferable(type:)
method with the PhotosPickerItem
object and using the resulting Image
on your SwiftUI interface.
import SwiftUI
import PhotosUI
struct ContentView: View {
@State private var avatarPhotoItem: PhotosPickerItem?
@State private var selectedImage: Image?
var body: some View {
VStack {
Spacer()
selectedImage?
.resizable()
.scaledToFit()
.clipShape(.rect(cornerRadius: 15))
.padding()
Spacer()
PhotosPicker("Select image",
selection: $avatarPhotoItem,
matching: .images)
}
.task(id: avatarPhotoItem) {
selectedImage = try? await avatarPhotoItem?
.loadTransferable(type: Image.self)
}
}
}
Showing the picked image on a SwiftUI app interface
In the example above, when a new image is selected a task is triggered and we load an Image
representation of the selected item to be displayed on the interface.