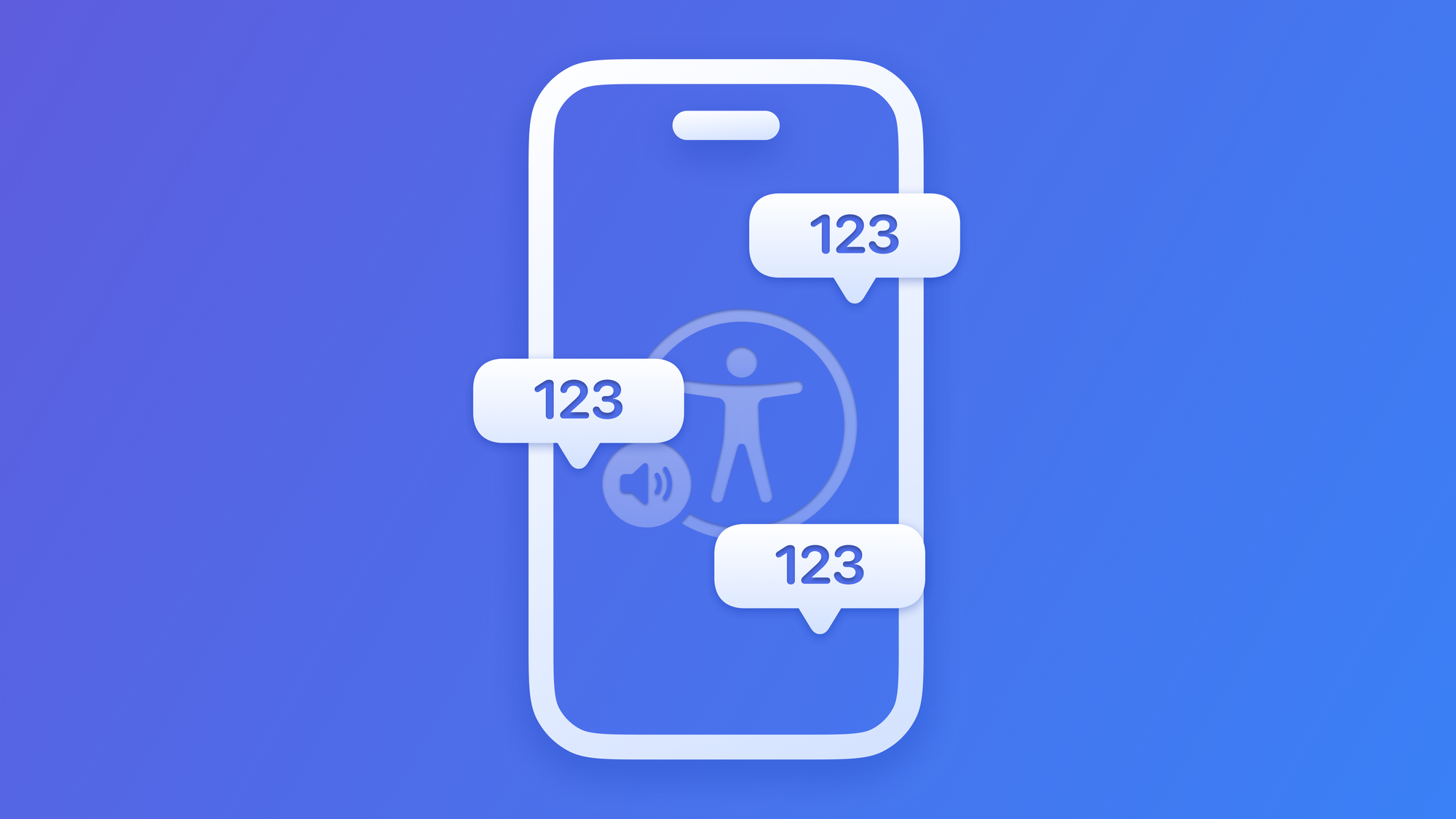
Preparing your App for VoiceOver: Accessibility Value
Ensure the interface elements of your app properly inform their current value for assistive technologies
In Preparing your App for VoiceOver: use Accessibility Label we discussed how to properly identify each component of the user interface for assistive APIs. In this article, we will cover another important accessibility attribute: accessibility value.
The accessibility value is the accessibility element’s current value, according to the official Apple Documentation.
For elements with changing content not described in their labels, it's important to set an accessibility value. Standard controls usually manage this, but for custom elements, you need to set this accessibility API properly.
Accessibility Values play a crucial role, especially with VoiceOver. When a user changes a control's value, VoiceOver instantly announces the new value avoiding repeated information.
How to use Accessibility values
UIKit and SwiftUI can often infer the value from native components. The slider component is a perfect example of how the accessibilityValue
is managed in both frameworks.
SwiftUI
You can use the .accessibilityValue()
modifier to provide an accessibility value for a view.
//Setting accessibility attributes on a Slider component in SwiftUI
Slider(value: $volume, in: 0...10, step: 1.0)
.accessibilityValue(String(format: "%.0f", volume))
.accessibilityLabel(Text("Volume Slider, the current value is:"))
Or you can use the Value field in the Accessibility section of the Attribute Inspector in the Xcode's right Inspectors panel once a component is selected.
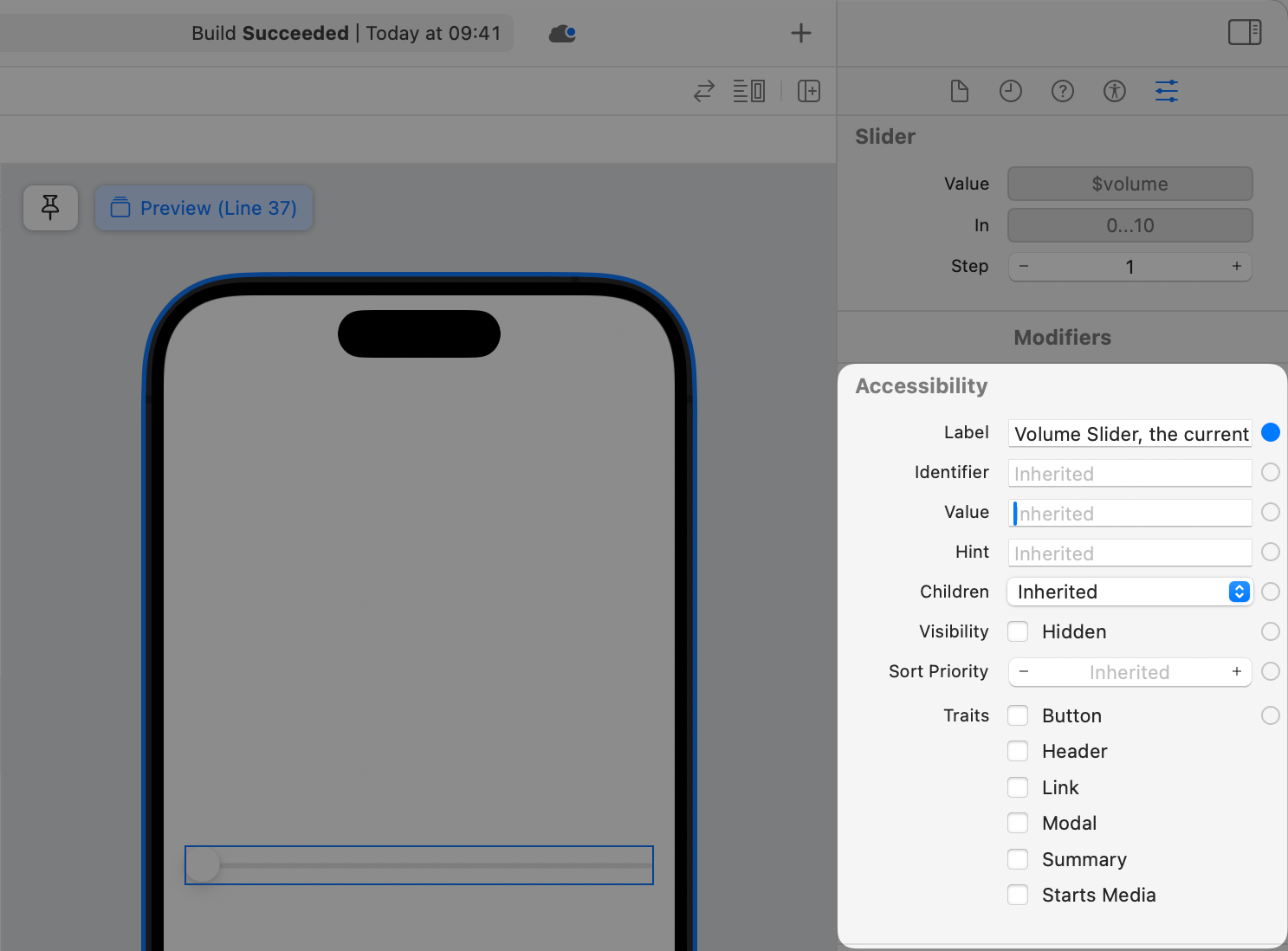
UIKit
You can use the accessibilityValue
property to set the accessibility value of the component.
class ViewController: UIViewController {
@IBOutlet weak var slider: UISlider!
override func viewDidLoad() {
super.viewDidLoad()
slider.minimumValue = 0
slider.maximumValue = 10
slider.value = 5
slider.accessibilityLabel = "Volume Slider, the current value is:"
}
// Setting accessibility attributes on a Slider component in UIKit
@IBAction func sliderChanged(_ sender: UISlider) {
slider.accessibilityValue = String(sender.value)
}
}
When to use it :
An accessibility element has a static label and a dynamic value
One example could be the mail app. In the Inbox row, the user has some emails to check. VoiceOver will say “Inbox” first because is the inferred accessibility label, and then it will say “x Unread Messages” which is the value associated with the row.
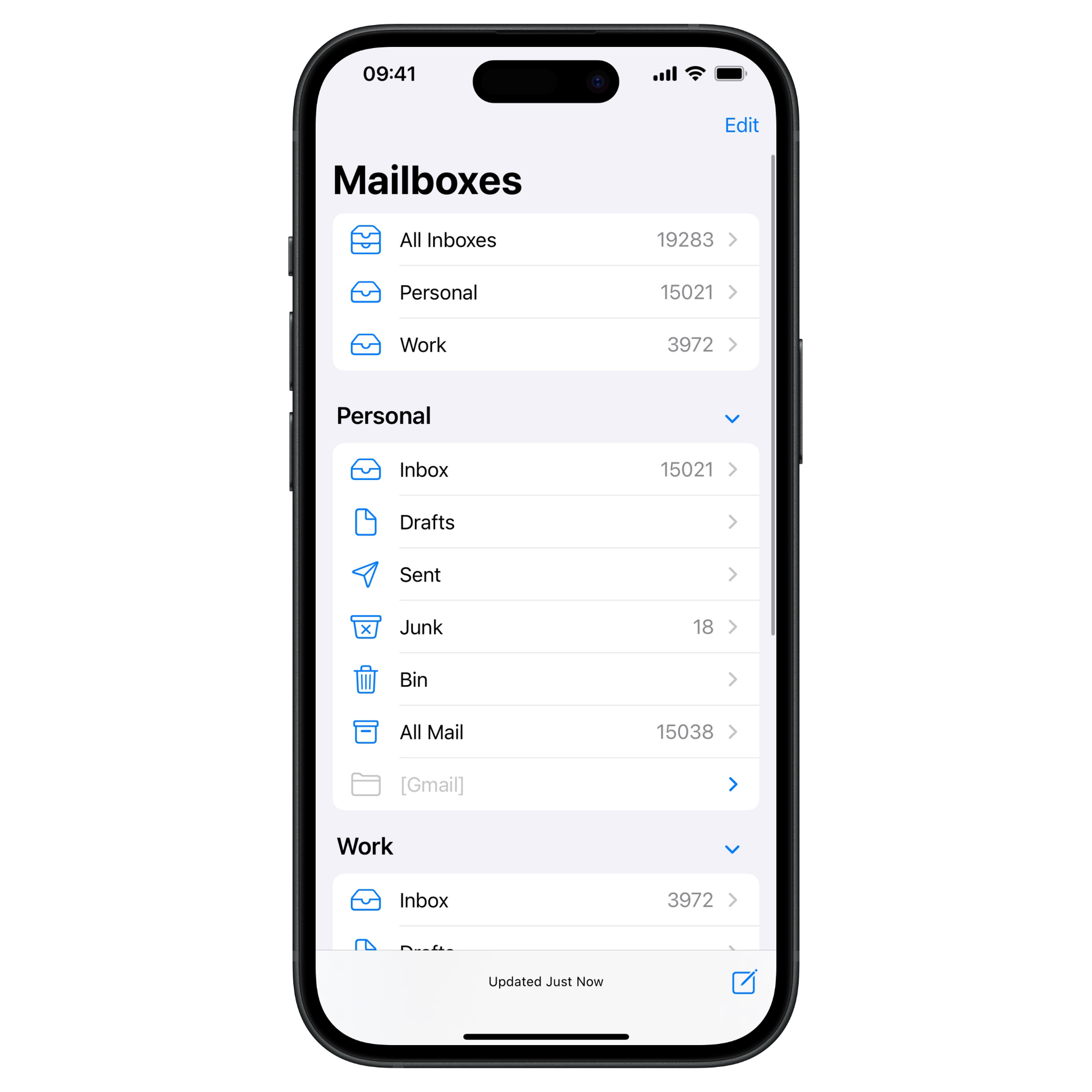
Navigation between different tabs
When you implement a TabView with the .tabViewStyle(.page)
modifier inside your app you can enhance the navigation experience with accessibility values. Let’s take a look at the Weather app.
When you select the Tab View controller interface element it will say “Current location: …. , city 1/…”. This is fundamental to give users information about which page is selected and the number of pages that are possible to interact with.
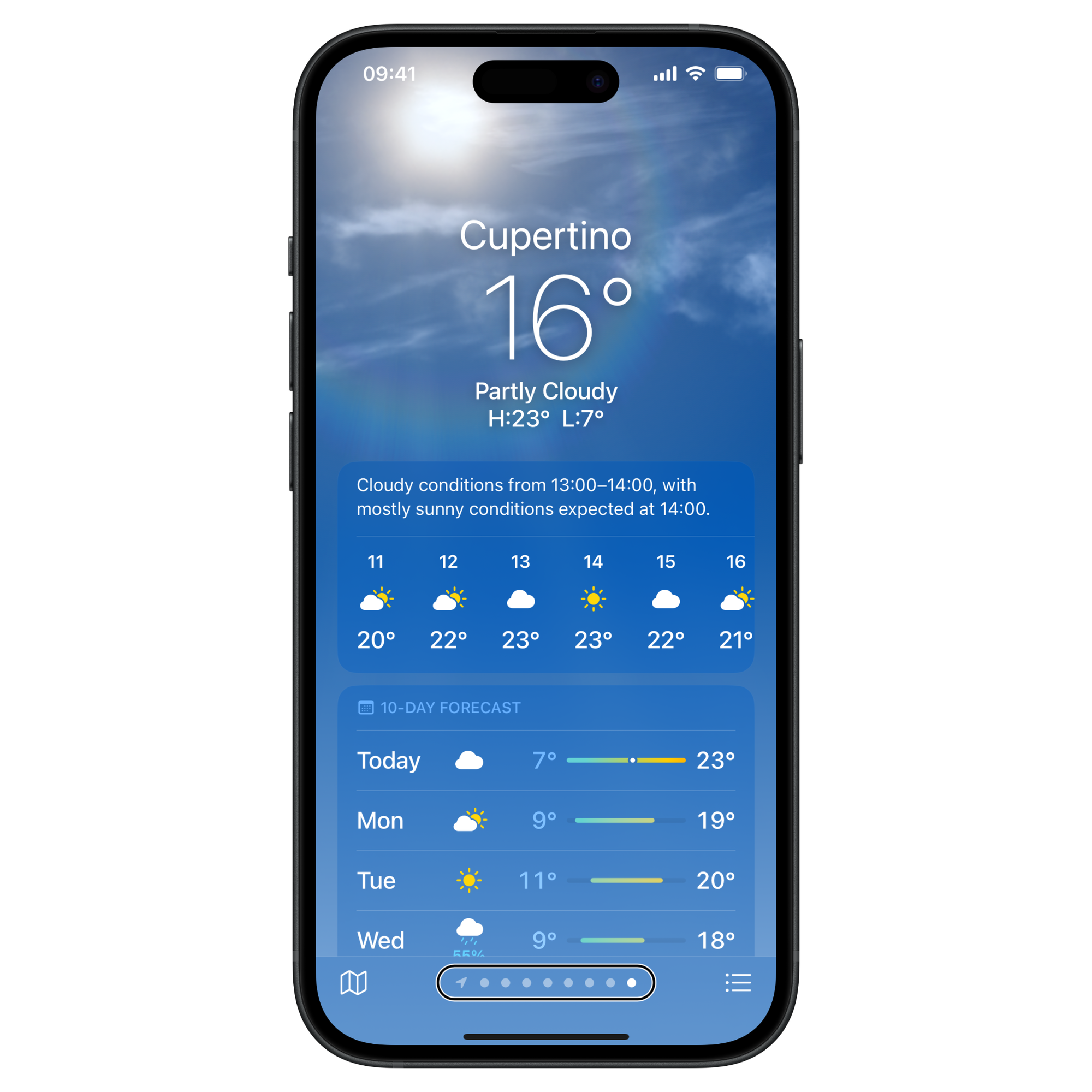
Update basic value
For a basic control, such as the Toggle, you can set the value to be read using the accessibilityValue
modifier. When VoiceOver reads a Toggle component, a double tap will announce only the current value of the toggle.
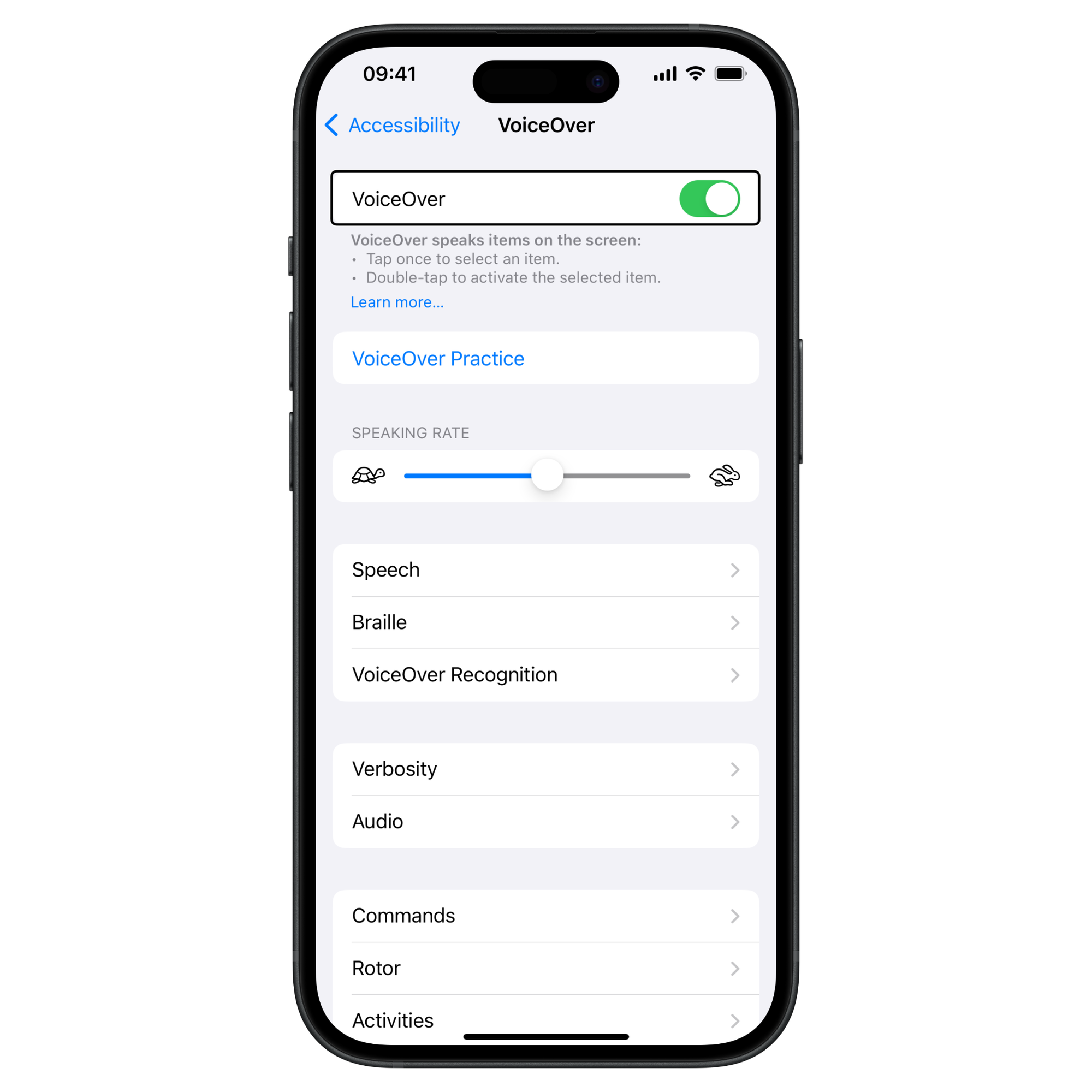
Placeholder text in Textfields
Use the accessibilityValue
modifier for placeholder text inside a Text Field component. Using accessibilityValue
ensures that VoiceOver conveys any changes as the user inputs information.
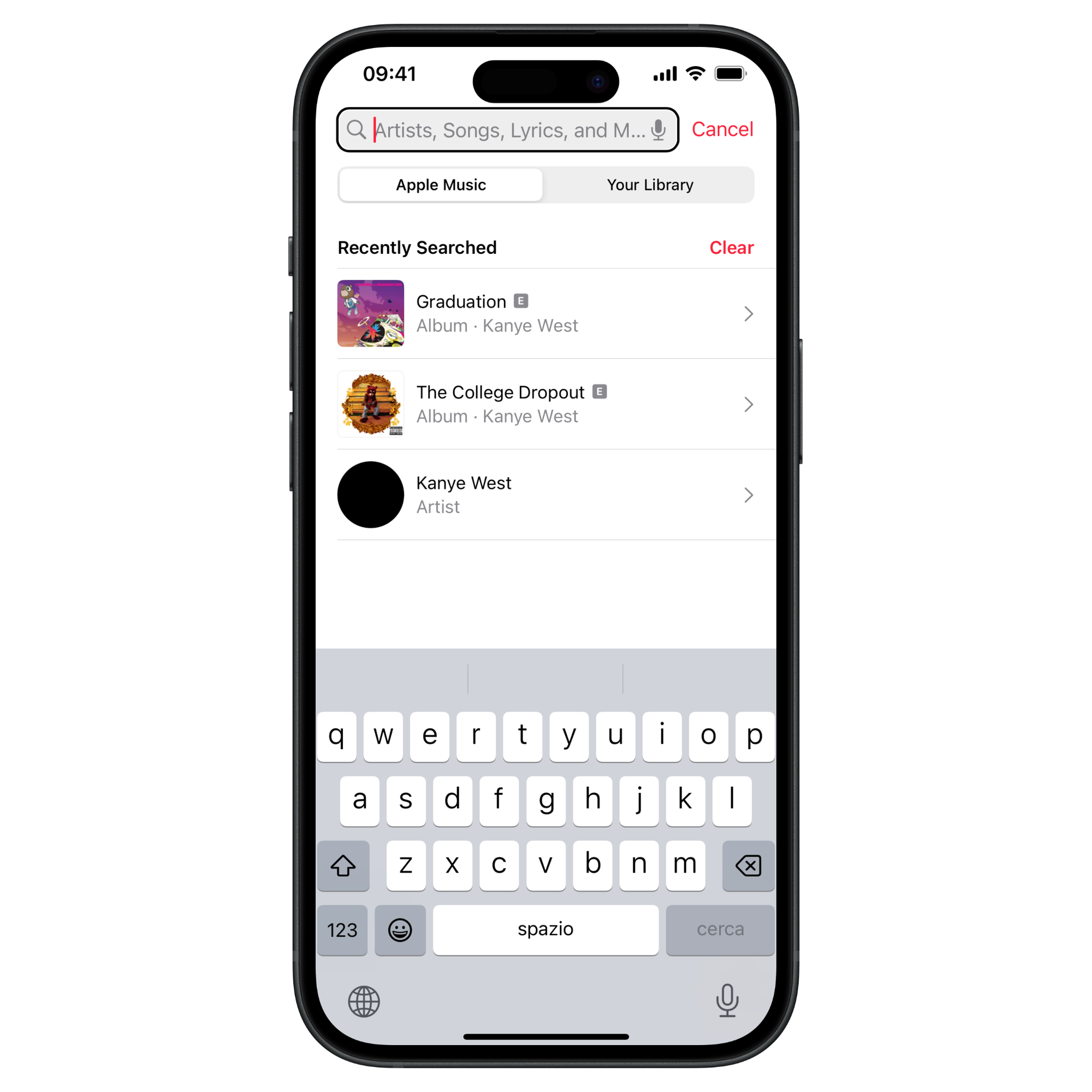
When not to use it
Avoid using accessibilityValue
when you need to provide additional guidance or contextual information for users. Instead, consider using the Accessibility Label or Hint attributes for controls that require more explanation.
WCAG Compliance
In the WCAG 2.2 (Web Content Accessibility Guidelines), the industry-standard accessibility reference, we can see the following guidelines:
Guideline 1.1 Text Alternatives
"Provide text alternatives for any non-text content so that it can be changed into other forms people need, such as large print, braille, speech, symbols or simpler language."
Success Criterion 4.1.2 Name, Role, Value
For all user interface components (including but not limited to: form elements, links and components generated by scripts), the name and role can be programmatically determined; states, properties, and values that can be set by the user can be programmatically set; and notification of changes to these items is available to user agents, including assistive technologies.