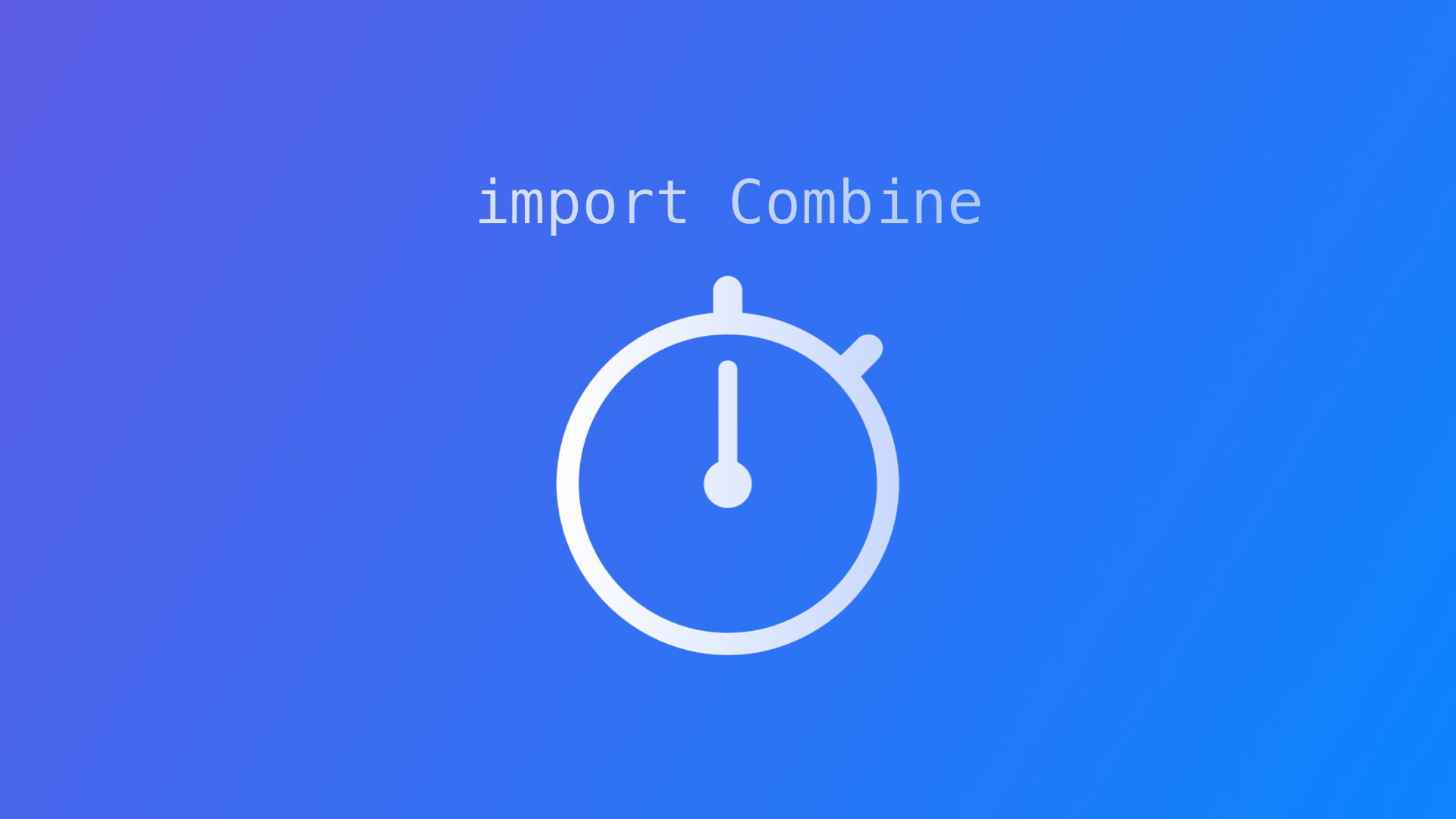
Combine: Working with Timers
With these code snippets you can learn about scheduling publishers in Combine with timers to modify their behavior.
This brief overview will demonstrate some basic features that may come in handy when working with publishers in Combine, Apple's framework to handle asynchronous events by combining event-processing operators. The Publisher protocol declares a type that transmits a sequence of values over time that subscribers can receive as input by adopting the Subscriber protocol.
Using a sequence as values to publish, Combine allows typical operators available in Swift to shape the values that will be published or received. For example, publishers can be used with timers to schedule their behavior.
Timers can be created using the RunLoop, for example with 3 second interval:
import Foundation
import Combine
let runLoop = RunLoop.main
let subscription = runLoop.schedule(after: runLoop.now, interval: .seconds(2) , tolerance: .milliseconds(100)) {
//what happens when timer is fired
print("Timer fired")
}
runLoop.schedule(after: .init(Date(timeIntervalSinceNow: 3.0))) {
subscription.cancel()
}
Alternatively, the Timer class can be utilized, resulting in a more reactive compositing of the timer. For example with a 1 second interval:
let timerSubscription = Timer.publish(every: 1.0, on: .main, in: .common)
.autoconnect()
.scan(0) { counter, _ in
counter + 1
}
.sink {
print($0)
}
Then, you can of course also use the DispatchQueue to schedule publishers, for example with a 2 second interval in this case:
let queue = DispatchQueue.main
let source = PassthroughSubject<Int, Never>()
var counter = 0
let cancellable = queue.schedule(after: queue.now, interval: .seconds(1)) {
source.send(counter)
counter += 1
}
let queueSubscription = source.sink {
print($0)
}
All in all, it's quite convenient to setup timers to schedule publisher behavior.
Where to go next?
If you are interested in knowing more about working with Combine and the various operators available to shape the sequence of values send by publishers and received by subscribers, check our other articles on Combine that will be released over the next days and weeks.
They will cover how to work with filters, sequence, and transformation operatators or timers, how to use the dataTaskPublisher, how to combine publishers as well as how to debug Combine messages, and much more.