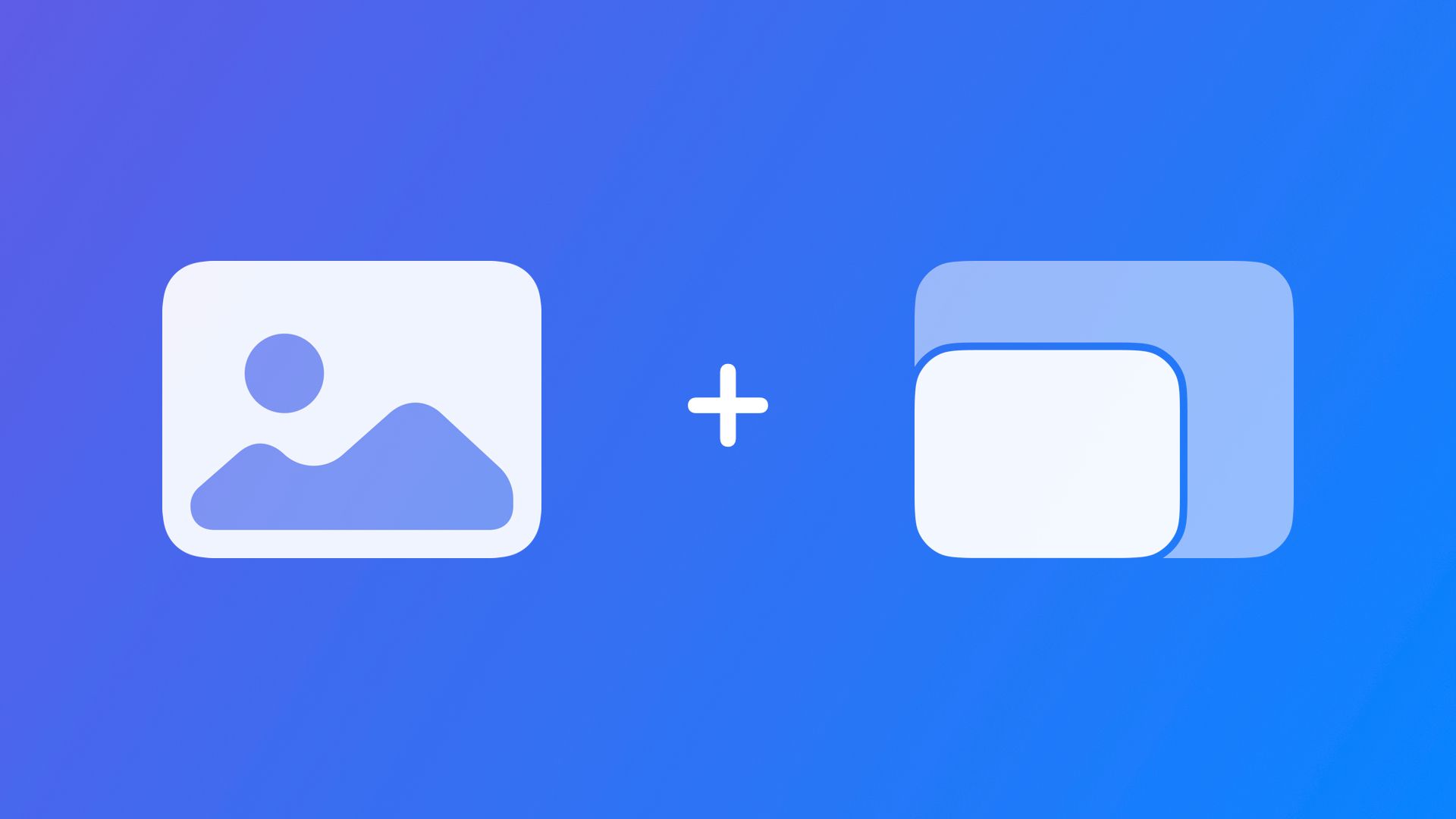
UIImage+Resize: Resizing an UIImage
With this UIImage extension you will be able to resize an UIImage to any size using a CGSize structure with width and height values as a parameter.
In some contexts it might be necessary to quickly resize an image to meet certain requirements, e.g. optimizing memory footprint when displaying images at actual sizes or when providing image data as input for a Core ML model.
When using UIImage fore the image data, the image can be conveniently resized with the following UIImage+Resize extension by providing width and height values as input parameters suing a CGSize structure.
import Foundation
import UIKit
extension UIImage {
func resizeImageTo(size: CGSize) -> UIImage? {
UIGraphicsBeginImageContextWithOptions(size, false, 0.0)
self.draw(in: CGRect(origin: CGPoint.zero, size: size))
let resizedImage = UIGraphicsGetImageFromCurrentImageContext()!
UIGraphicsEndImageContext()
return resizedImage
}
}
In context of using an UIImage as an input for a Core ML model with the Vision framework, the image has to comply to specific requirements that relate to both the resolution of the image and also the datatype itself. As the Vision framework is using data formats from Core Graphics, Core Image or Core Video, the UIImage also has to be converted to any of those parameters. While UIImage provides convenience instance properties for CGImage and CIImage, converting the UIImage to a Core Video Pixel Buffer can be achieved with the UIImage+CVPixelBuffer extensions.
For an extension to UIImage that combines both the resizing and conversion to CVPixelBuffer, also consider the UIImage+Resize+CVPixelbuffer extension.
This reference is part of a series of articles derived from the presentation Creating Machine Learning Models with Create ML presented as a one time event at the Swift Heroes 2021 Digital Conference on April 16th, 2021.
Where to go next?
If you are interested into knowing more about uses cases for resizing UIImage you can check other tutorials on:
- Use an Object Detection Machine Learning Model in an iOS App using SwiftUI
- Use an Object Detection Machine Learning Model in Swift Playgrounds
You want to know more? There is more to see...
Recommended Content provided by Apple:
For a deeper dive on the topic of UIImage and how to manage image data in UIKIt, you can explore these materials released by Apple in the official Developer Documentation:
- Class: UIImage
- Instance Method: resizableImage(withCapInsets:)
- Class: UIImageView