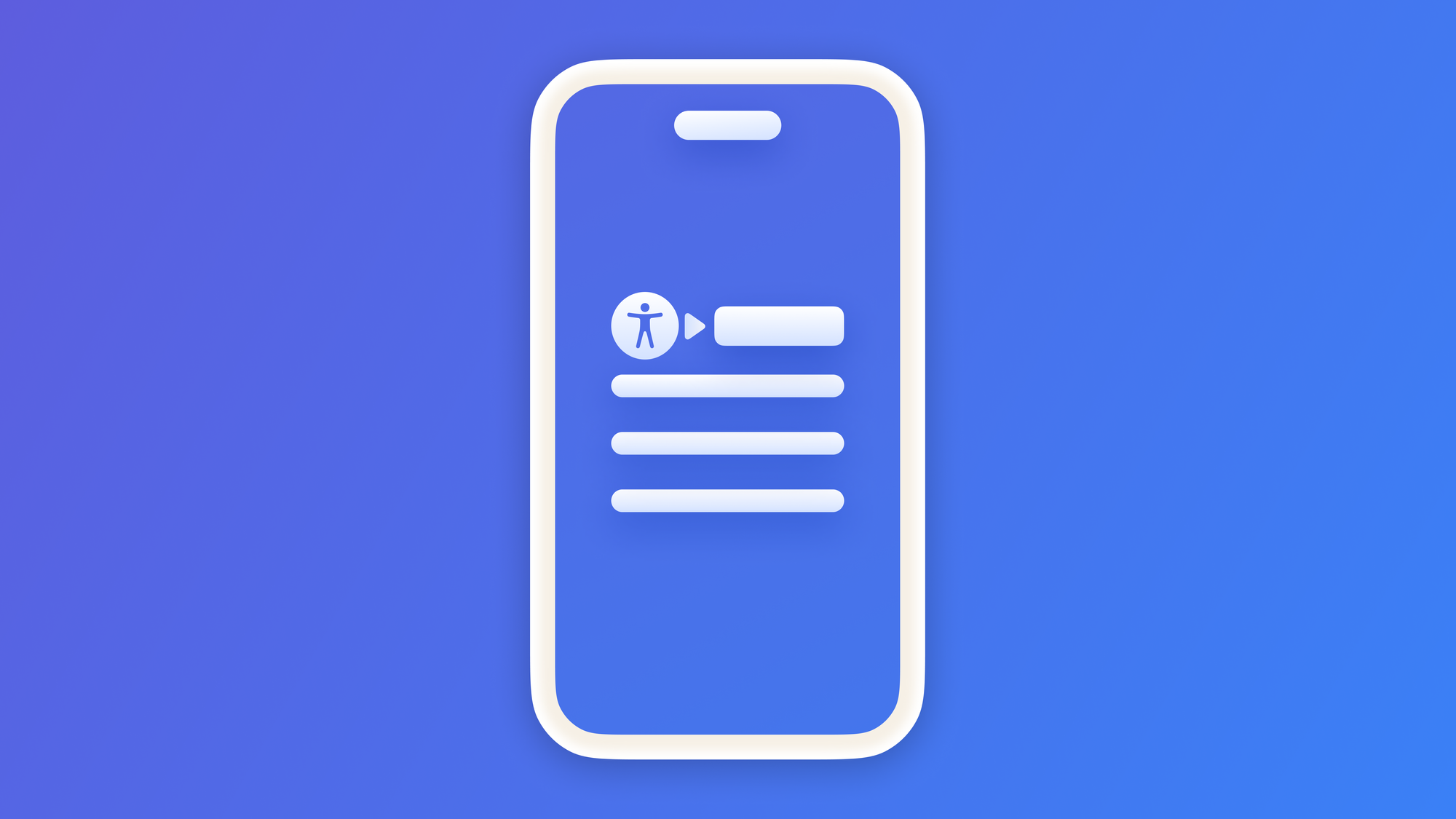
Preparing your App for VoiceOver: customizing the Sort Priority
Ensure the correct reading order of your interface elements by VoiceOver.
In Ensure Visual Accessibility: Using Typography we discussed how, through the use of appropriate fonts, sizes, and styles, we can create a visual hierarchy that guides the user's eyes from one level of importance to another.
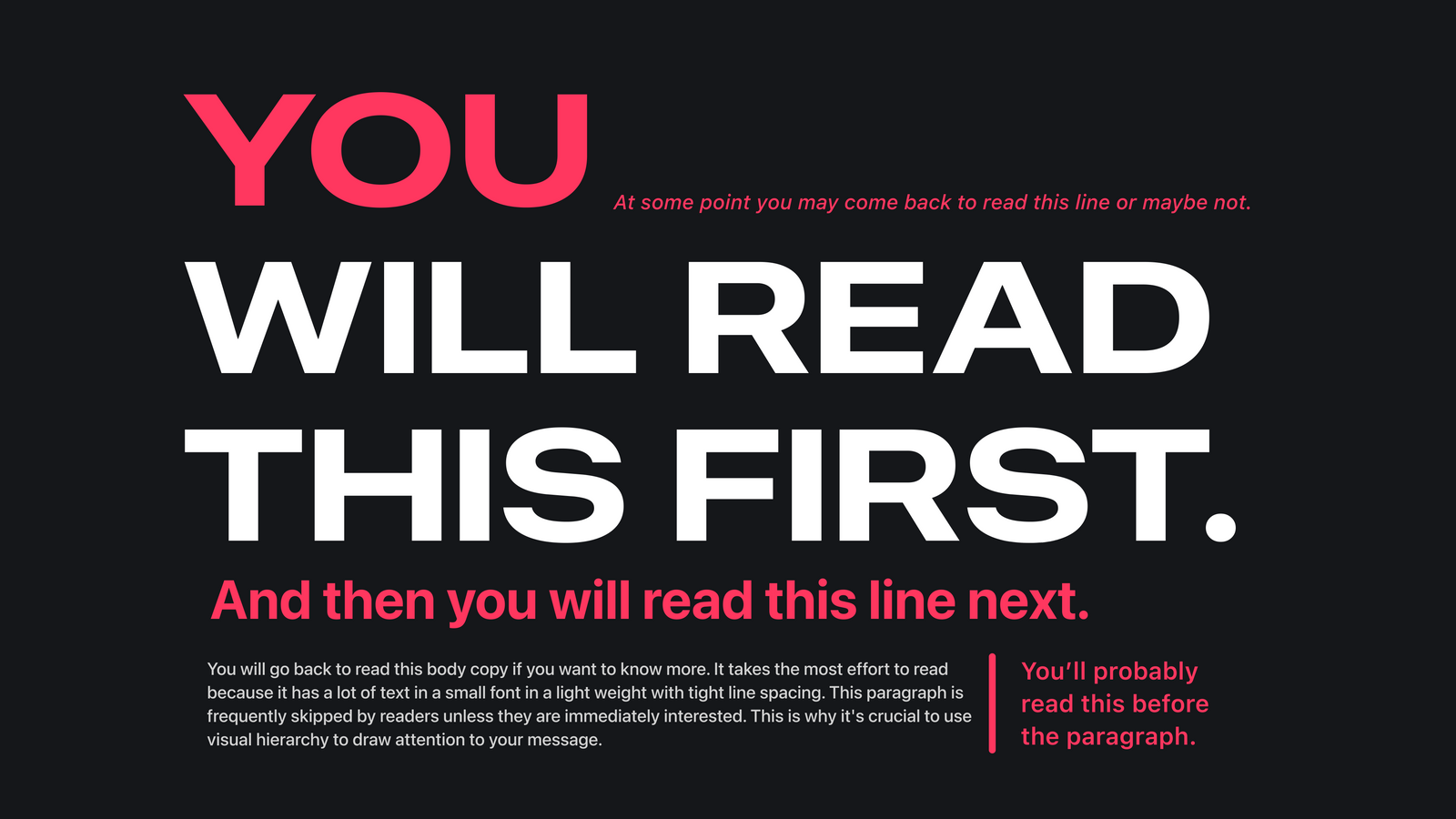
Sometimes we may want to create the the same hierarchy for users that do not rely on vision but on assistive technologies to perceive the interface.
In Understanding the Accessible User Interface we said that the Accessible User Interface (AUI), also known as the Accessibility Tree, and the standard User Interface (UI) often diverge significantly regarding hierarchy.
In fact, by default, assistive technologies will create the Accessible Tree following the natural reading direction and top to bottom. If you have set up your device in English, Voice Over will read the Accessibility Elements of your interface from left to right and top to bottom since English is a Left-to-Right (LTR) Language.
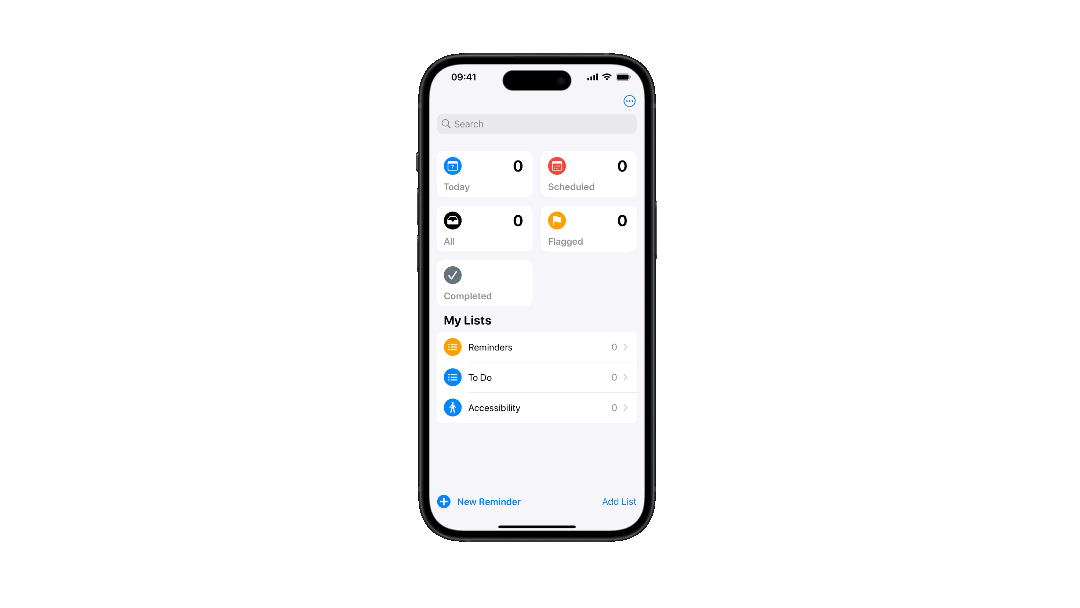
Modifying the order in which assistive technologies access elements is crucial for establishing a user-friendly navigation experience.
The "WWDC23 highlights" article card in the Developer App
The Stopwatch actions in the Clock App
How to customize the Sort Priority
Both UIKit and SwiftUI provide ways to customize the priority of each element of the Accessibility Tree.
UIKit
You can use the accessibilityElements
property of the view to define an array of accessible elements. Assistive technologies will access the elements following the order of the array.
//Customize the Accessibility Elements order in UIKit
import UIKit
class AccessibleViewController: UIViewController {
@IBOutlet weak var image: UIImageView!
@IBOutlet weak var firstLabel: UILabel!
@IBOutlet weak var secondLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
image.image = UIImage(systemName: "accessibility")
firstLabel.text = "Hello, Accessibility"
secondLabel.text = "Customize the Accessibility Elements order"
self.view.accessibilityElements = [secondLabel!,firstLabel!, image!]
}
}
SwiftUI
You can use the accessibilitySortPriority(_:)
modifier with a sort priority parameter.
Sets the sort priority order for this view’s accessibility element, relative to other elements at the same level.
Apple Developer Documentation
The higher the parameter, the higher the priority for the assistive API.
//Customize the Accessibility Elements order in SwiftUI
VStack {
Image(systemName: "accessibility.fill")
.foregroundStyle(.tint)
.imageScale(.large)
.accessibilityHidden(true)
Text("Hello, Accessibility")
.accessibilitySortPriority(1)
Text("Using the accessibilitySortPriority modifier")
.accessibilitySortPriority(2)
}
Or you can use the Sort Priority field in the Accessibility section of the Attribute Inspector in the Xcode's Inspectors panel once a component is selected.
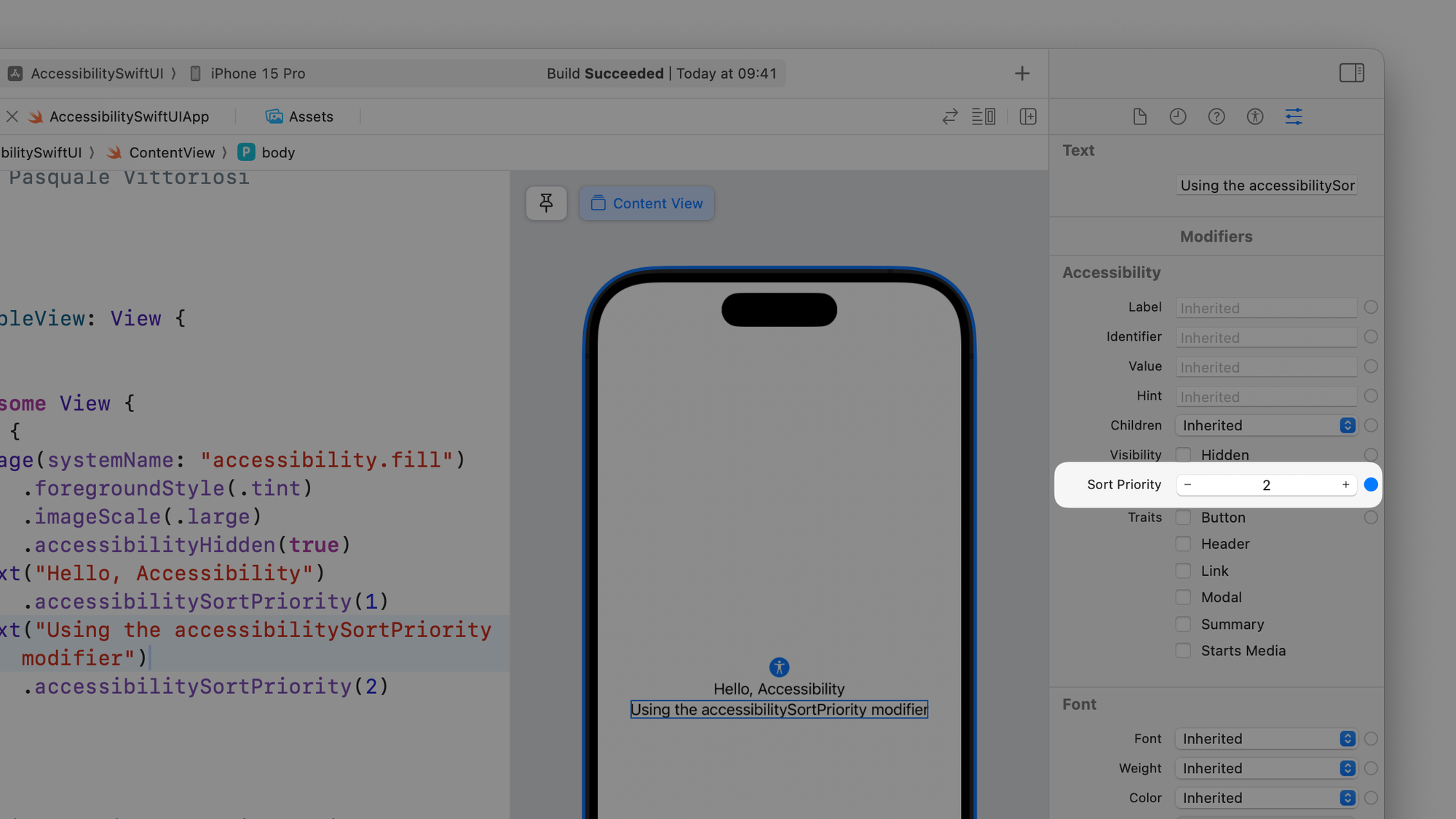
When to provide a custom sorting to the interface elements
You have a complex view hierarchy or custom layouts
If your view hierarchy is structured in a way that VoiceOver can't infer the correct order to read the interface elements, you should change the sorting priority of the interface elements to ensure they are read in a way that the user can navigate properly through the interface.
Visual order differs from reading order
Sometimes, the visual layout of elements on the screen doesn't match the intended reading order. When that happens you must inform the correct sorting priority to VoiceOver.
When not to provide a custom sorting to the interface elements
Give priority to what you want the user to access first
The priority in sorting elements should be based on what makes the most semantic sense to users. You should only do it if it enhances the logical navigation of your app.
The order in which elements are sorted should be chosen to align with what makes the most semantic sense to the user, which usually is the reading direction and top to bottom, ensuring a navigation experience that feels logical and intuitive.
Altering the sequence in which accessibility elements receive focus through assistive technology is a significant decision that developers should approach with careful consideration.
Compliance with Web Content Accessibility Guidelines
In the WCAG 2.2 (Web Content Accessibility Guidelines), the industry-standard accessibility reference, we can see the following guidelines:
Guideline 1.3 Adaptable
Create content that can be presented in different ways (for example simpler layout) without losing information or structure.
One of the success criteria is:
Success Criterion 1.3.2 Meaningful Sequence
When the sequence in which content is presented affects its meaning, a correct reading sequence can be programmatically determined.
but also:
Guideline 2.4 Navigable
Provide ways to help users navigate, find content, and determine where they are.
and related success criteria:
Success Criterion 2.4.3 Focus Order
If a Web page can be navigated sequentially and the navigation sequences affect meaning or operation, focusable components receive focus in an order that preserves meaning and operability.
You can comply with these guidelines by providing the correct reading order of the interface elements for the assistive technologies in your app.
Related Articles
- Understanding the Accessible User Interface
- Preparing your App for VoiceOver: use Accessibility Label
- Preparing your App for VoiceOver: Labels, Values and Hints
- Preparing your App for VoiceOver: Accessibility Traits
- Preparing your App for VoiceOver: Hiding Elements from the Accessible Interface
- Testing your app's accessibility with the Accessibility Inspector